mirror of
https://github.com/u-boot/u-boot.git
synced 2025-04-16 18:04:48 +00:00
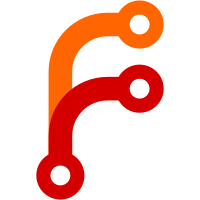
Add support for PHYTEC phyCORE-AM62A7 SoM. Supported features: - 2GB LPDDR4 RAM - eMMC - External SD - Ethernet - debug UART Signed-off-by: Garrett Giordano <ggiordano@phytec.com> Reviewed-by: Wadim Egorov <w.egorov@phytec.de> Reviewed-by: Tom Rini <trini@konsulko.com> Reviewed-by: Bryan Brattlof <bb@ti.com>
64 lines
1.4 KiB
C
64 lines
1.4 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later OR MIT
|
|
/*
|
|
* Copyright (C) 2024 PHYTEC America LLC
|
|
* Author: Garrett Giordano <ggiordano@phytec.com>
|
|
*/
|
|
|
|
#include <asm/arch/hardware.h>
|
|
#include <asm/io.h>
|
|
#include <spl.h>
|
|
#include <fdt_support.h>
|
|
|
|
#include "../common/am6_som_detection.h"
|
|
|
|
int board_init(void)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
int dram_init(void)
|
|
{
|
|
return fdtdec_setup_mem_size_base();
|
|
}
|
|
|
|
int dram_init_banksize(void)
|
|
{
|
|
return fdtdec_setup_memory_banksize();
|
|
}
|
|
|
|
#define CTRLMMR_USB0_PHY_CTRL 0x43004008
|
|
#define CTRLMMR_USB1_PHY_CTRL 0x43004018
|
|
#define CORE_VOLTAGE 0x80000000
|
|
|
|
#ifdef CONFIG_SPL_BOARD_INIT
|
|
void spl_board_init(void)
|
|
{
|
|
u32 val;
|
|
|
|
/* Set USB0 PHY core voltage to 0.85V */
|
|
val = readl(CTRLMMR_USB0_PHY_CTRL);
|
|
val &= ~(CORE_VOLTAGE);
|
|
writel(val, CTRLMMR_USB0_PHY_CTRL);
|
|
|
|
/* Set USB1 PHY core voltage to 0.85V */
|
|
val = readl(CTRLMMR_USB1_PHY_CTRL);
|
|
val &= ~(CORE_VOLTAGE);
|
|
writel(val, CTRLMMR_USB1_PHY_CTRL);
|
|
|
|
if (IS_ENABLED(CONFIG_SPL_ETH))
|
|
/* Init DRAM size for R5/A53 SPL */
|
|
dram_init_banksize();
|
|
|
|
/* We have 32k crystal, so lets enable it */
|
|
val = readl(MCU_CTRL_LFXOSC_CTRL);
|
|
val &= ~(MCU_CTRL_LFXOSC_32K_DISABLE_VAL);
|
|
writel(val, MCU_CTRL_LFXOSC_CTRL);
|
|
/* Add any TRIM needed for the crystal here.. */
|
|
/* Make sure to mux up to take the SoC 32k from the crystal */
|
|
writel(MCU_CTRL_DEVICE_CLKOUT_LFOSC_SELECT_VAL,
|
|
MCU_CTRL_DEVICE_CLKOUT_32K_CTRL);
|
|
|
|
/* Init DRAM size for R5/A53 SPL */
|
|
dram_init_banksize();
|
|
}
|
|
#endif
|