mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-23 13:36:05 +00:00
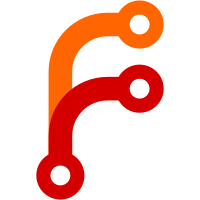
Enforce full include path for includes. Deprecate old paths. The following folders inside include/lib have been left unchanged: - include/lib/cpus/${ARCH} - include/lib/el3_runtime/${ARCH} The reason for this change is that having a global namespace for includes isn't a good idea. It defeats one of the advantages of having folders and it introduces problems that are sometimes subtle (because you may not know the header you are actually including if there are two of them). For example, this patch had to be created because two headers were called the same way:e0ea0928d5
("Fix gpio includes of mt8173 platform to avoid collision."). More recently, this patch has had similar problems:46f9b2c3a2
("drivers: add tzc380 support"). This problem was introduced in commit4ecca33988
("Move include and source files to logical locations"). At that time, there weren't too many headers so it wasn't a real issue. However, time has shown that this creates problems. Platforms that want to preserve the way they include headers may add the removed paths to PLAT_INCLUDES, but this is discouraged. Change-Id: I39dc53ed98f9e297a5966e723d1936d6ccf2fc8f Signed-off-by: Antonio Nino Diaz <antonio.ninodiaz@arm.com>
109 lines
2.9 KiB
C
109 lines
2.9 KiB
C
/*
|
|
* Copyright (c) 2015-2017, Renesas Electronics Corporation. All rights reserved.
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
|
|
#include <arch_helpers.h>
|
|
#include <common/bl_common.h>
|
|
#include <common/debug.h>
|
|
#include <common/runtime_svc.h>
|
|
#include <drivers/arm/gicv2.h>
|
|
#include <lib/mmio.h>
|
|
|
|
#include "rcar_def.h"
|
|
|
|
#define SWDT_ERROR_ID (1024U)
|
|
#define SWDT_ERROR_TYPE (16U)
|
|
#define SWDT_CHAR_MAX (13U)
|
|
|
|
extern void rcar_swdt_release(void);
|
|
|
|
void bl2_interrupt_error_id(uint32_t int_id)
|
|
{
|
|
ERROR("\n");
|
|
if (int_id >= SWDT_ERROR_ID) {
|
|
ERROR("Unhandled exception occurred.\n");
|
|
ERROR(" Exception type = FIQ_SP_ELX\n");
|
|
panic();
|
|
}
|
|
|
|
/* Clear the interrupt request */
|
|
gicv2_end_of_interrupt((uint32_t) int_id);
|
|
rcar_swdt_release();
|
|
ERROR("Unhandled exception occurred.\n");
|
|
ERROR(" Exception type = FIQ_SP_ELX\n");
|
|
ERROR(" SPSR_EL1 = 0x%x\n", (uint32_t) read_spsr_el1());
|
|
ERROR(" ELR_EL1 = 0x%x\n", (uint32_t) read_elr_el1());
|
|
ERROR(" ESR_EL1 = 0x%x\n", (uint32_t) read_esr_el1());
|
|
ERROR(" FAR_EL1 = 0x%x\n", (uint32_t) read_far_el1());
|
|
ERROR("\n");
|
|
panic();
|
|
}
|
|
|
|
void bl2_interrupt_error_type(uint32_t ex_type)
|
|
{
|
|
const uint8_t interrupt_ex[SWDT_ERROR_TYPE][SWDT_CHAR_MAX] = {
|
|
"SYNC SP EL0",
|
|
"IRQ SP EL0",
|
|
"FIQ SP EL0",
|
|
"SERR SP EL0",
|
|
"SYNC SP ELx",
|
|
"IRQ SP ELx",
|
|
"FIQ SP ELx",
|
|
"SERR SP ELx",
|
|
"SYNC AARCH64",
|
|
"IRQ AARCH64",
|
|
"FIQ AARCH64",
|
|
"SERR AARCH64",
|
|
"SYNC AARCH32",
|
|
"IRQ AARCH32",
|
|
"FIQ AARCH32",
|
|
"SERR AARCH32"
|
|
};
|
|
char msg[128];
|
|
|
|
/* Clear the interrupt request */
|
|
if (ex_type >= SWDT_ERROR_TYPE) {
|
|
ERROR("\n");
|
|
ERROR("Unhandled exception occurred.\n");
|
|
ERROR(" Exception type = Unknown (%d)\n", ex_type);
|
|
goto loop;
|
|
}
|
|
|
|
rcar_swdt_release();
|
|
ERROR("\n");
|
|
ERROR("Unhandled exception occurred.\n");
|
|
snprintf(msg, sizeof(msg), " Exception type = %s\n",
|
|
&interrupt_ex[ex_type][0]);
|
|
ERROR("%s", msg);
|
|
switch (ex_type) {
|
|
case SYNC_EXCEPTION_SP_ELX:
|
|
ERROR(" SPSR_EL1 = 0x%x\n", (uint32_t) read_spsr_el1());
|
|
ERROR(" ELR_EL1 = 0x%x\n", (uint32_t) read_elr_el1());
|
|
ERROR(" ESR_EL1 = 0x%x\n", (uint32_t) read_esr_el1());
|
|
ERROR(" FAR_EL1 = 0x%x\n", (uint32_t) read_far_el1());
|
|
break;
|
|
case IRQ_SP_ELX:
|
|
ERROR(" SPSR_EL1 = 0x%x\n", (uint32_t) read_spsr_el1());
|
|
ERROR(" ELR_EL1 = 0x%x\n", (uint32_t) read_elr_el1());
|
|
ERROR(" IAR_EL1 = 0x%x\n", gicv2_acknowledge_interrupt());
|
|
break;
|
|
case FIQ_SP_ELX:
|
|
ERROR(" SPSR_EL1 = 0x%x\n", (uint32_t) read_spsr_el1());
|
|
ERROR(" ELR_EL1 = 0x%x\n", (uint32_t) read_elr_el1());
|
|
ERROR(" IAR_EL1 = 0x%x\n", gicv2_acknowledge_interrupt());
|
|
break;
|
|
case SERROR_SP_ELX:
|
|
ERROR(" SPSR_EL1 = 0x%x\n", (uint32_t) read_spsr_el1());
|
|
ERROR(" ELR_EL1 = 0x%x\n", (uint32_t) read_elr_el1());
|
|
ERROR(" ESR_EL1 = 0x%x\n", (uint32_t) read_esr_el1());
|
|
ERROR(" FAR_EL1 = 0x%x\n", (uint32_t) read_far_el1());
|
|
break;
|
|
default:
|
|
break;
|
|
}
|
|
loop:
|
|
ERROR("\n");
|
|
panic();
|
|
}
|