mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-08 05:43:53 +00:00
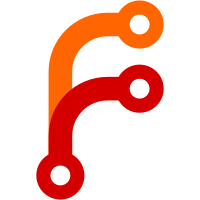
Enforce full include path for includes. Deprecate old paths. The following folders inside include/lib have been left unchanged: - include/lib/cpus/${ARCH} - include/lib/el3_runtime/${ARCH} The reason for this change is that having a global namespace for includes isn't a good idea. It defeats one of the advantages of having folders and it introduces problems that are sometimes subtle (because you may not know the header you are actually including if there are two of them). For example, this patch had to be created because two headers were called the same way:e0ea0928d5
("Fix gpio includes of mt8173 platform to avoid collision."). More recently, this patch has had similar problems:46f9b2c3a2
("drivers: add tzc380 support"). This problem was introduced in commit4ecca33988
("Move include and source files to logical locations"). At that time, there weren't too many headers so it wasn't a real issue. However, time has shown that this creates problems. Platforms that want to preserve the way they include headers may add the removed paths to PLAT_INCLUDES, but this is discouraged. Change-Id: I39dc53ed98f9e297a5966e723d1936d6ccf2fc8f Signed-off-by: Antonio Nino Diaz <antonio.ninodiaz@arm.com>
133 lines
3.4 KiB
ArmAsm
133 lines
3.4 KiB
ArmAsm
/*
|
|
* Copyright (c) 2013-2018, ARM Limited and Contributors. All rights reserved.
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
|
|
#include <arch.h>
|
|
#include <asm_macros.S>
|
|
#include <common/bl_common.h>
|
|
|
|
|
|
.globl bl2_entrypoint
|
|
|
|
|
|
|
|
func bl2_entrypoint
|
|
/*---------------------------------------------
|
|
* Save arguments x0 - x3 from BL1 for future
|
|
* use.
|
|
* ---------------------------------------------
|
|
*/
|
|
mov x20, x0
|
|
mov x21, x1
|
|
mov x22, x2
|
|
mov x23, x3
|
|
|
|
/* ---------------------------------------------
|
|
* Set the exception vector to something sane.
|
|
* ---------------------------------------------
|
|
*/
|
|
adr x0, early_exceptions
|
|
msr vbar_el1, x0
|
|
isb
|
|
|
|
/* ---------------------------------------------
|
|
* Enable the SError interrupt now that the
|
|
* exception vectors have been setup.
|
|
* ---------------------------------------------
|
|
*/
|
|
msr daifclr, #DAIF_ABT_BIT
|
|
|
|
/* ---------------------------------------------
|
|
* Enable the instruction cache, stack pointer
|
|
* and data access alignment checks
|
|
* ---------------------------------------------
|
|
*/
|
|
mov x1, #(SCTLR_I_BIT | SCTLR_A_BIT | SCTLR_SA_BIT)
|
|
mrs x0, sctlr_el1
|
|
orr x0, x0, x1
|
|
msr sctlr_el1, x0
|
|
isb
|
|
|
|
/* ---------------------------------------------
|
|
* Invalidate the RW memory used by the BL2
|
|
* image. This includes the data and NOBITS
|
|
* sections. This is done to safeguard against
|
|
* possible corruption of this memory by dirty
|
|
* cache lines in a system cache as a result of
|
|
* use by an earlier boot loader stage.
|
|
* ---------------------------------------------
|
|
*/
|
|
adr x0, __RW_START__
|
|
adr x1, __RW_END__
|
|
sub x1, x1, x0
|
|
bl inv_dcache_range
|
|
|
|
/* ---------------------------------------------
|
|
* Zero out NOBITS sections. There are 2 of them:
|
|
* - the .bss section;
|
|
* - the coherent memory section.
|
|
* ---------------------------------------------
|
|
*/
|
|
adrp x0, __BSS_START__
|
|
add x0, x0, :lo12:__BSS_START__
|
|
adrp x1, __BSS_END__
|
|
add x1, x1, :lo12:__BSS_END__
|
|
sub x1, x1, x0
|
|
bl zeromem
|
|
|
|
#if USE_COHERENT_MEM
|
|
adrp x0, __COHERENT_RAM_START__
|
|
add x0, x0, :lo12:__COHERENT_RAM_START__
|
|
adrp x1, __COHERENT_RAM_END_UNALIGNED__
|
|
add x1, x1, :lo12:__COHERENT_RAM_END_UNALIGNED__
|
|
sub x1, x1, x0
|
|
bl zeromem
|
|
#endif
|
|
|
|
/* --------------------------------------------
|
|
* Allocate a stack whose memory will be marked
|
|
* as Normal-IS-WBWA when the MMU is enabled.
|
|
* There is no risk of reading stale stack
|
|
* memory after enabling the MMU as only the
|
|
* primary cpu is running at the moment.
|
|
* --------------------------------------------
|
|
*/
|
|
bl plat_set_my_stack
|
|
|
|
/* ---------------------------------------------
|
|
* Initialize the stack protector canary before
|
|
* any C code is called.
|
|
* ---------------------------------------------
|
|
*/
|
|
#if STACK_PROTECTOR_ENABLED
|
|
bl update_stack_protector_canary
|
|
#endif
|
|
|
|
/* ---------------------------------------------
|
|
* Perform early platform setup & platform
|
|
* specific early arch. setup e.g. mmu setup
|
|
* ---------------------------------------------
|
|
*/
|
|
mov x0, x20
|
|
mov x1, x21
|
|
mov x2, x22
|
|
mov x3, x23
|
|
bl bl2_early_platform_setup2
|
|
|
|
bl bl2_plat_arch_setup
|
|
|
|
/* ---------------------------------------------
|
|
* Jump to main function.
|
|
* ---------------------------------------------
|
|
*/
|
|
bl bl2_main
|
|
|
|
/* ---------------------------------------------
|
|
* Should never reach this point.
|
|
* ---------------------------------------------
|
|
*/
|
|
no_ret plat_panic_handler
|
|
|
|
endfunc bl2_entrypoint
|