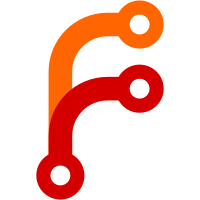
Added : Set as avatar code Added : Avatar Collection for user Added : new param avatar_collection in get_collections() method Fixed : crop value in thumbs_dimensions Added : is_avatar param in get_photos() method Added : photo_dimensions filter in generate_photos() method Fixed : insert_photo() method Moved : insert_photo_colors in insert_photo() method from view_item.php page Updated : get_image_type() method Updated : getFileSmarty() method Added : An anchor in getFileSmarty() 'photo_thumb' Fixed : cropping cases in resizer.class.php Updated : $Upload->upload_user_file to upload_new_avatar Removed : cb_create_user_avatar_collection() from common.php Registered : delete_photo_avatar() function @delete_photo Added : validate_image_file() function. It uses our old image.class.php method Added : get_mature_thumb() function. It has three parameters, object_array, size, output. You can also register custom thumb @mature_thumb Added : Few new functions for photos. get_all_custom_size_photos(), get_photo_dimensions(), clean_custom_photo_size(), get_avatar_photos() and is_photo_viewable() Added : new js function, displayConfirm(). It accepts four parameters. id( modal box id), confirmMessage( modal box body), onConfirm( either url or function ) and heading(modal box header)
160 lines
No EOL
3.3 KiB
PHP
160 lines
No EOL
3.3 KiB
PHP
<?php
|
|
/*
|
|
***************************************************************
|
|
| Copyright (c) 2007-2010 Clip-Bucket.com. All rights reserved.
|
|
| @ Author : ArslanHassan
|
|
| @ Software : ClipBucket , © PHPBucket.com
|
|
****************************************************************
|
|
*/
|
|
|
|
define("THIS_PAGE","edit_account");
|
|
|
|
require 'includes/config.inc.php';
|
|
$userquery->logincheck();
|
|
|
|
//Updating Profile
|
|
if(isset($_POST['update_profile']))
|
|
{
|
|
$array = $_POST;
|
|
$array['userid'] = userid();
|
|
$userquery->update_user($array);
|
|
}
|
|
|
|
//Updating Avatar
|
|
if(isset($_POST['update_avatar_bg']))
|
|
{
|
|
$array = $_POST;
|
|
$array['userid'] = userid();
|
|
$userquery->update_user_avatar_bg($array);
|
|
}
|
|
|
|
//Changing Email
|
|
if(isset($_POST['change_email']))
|
|
{
|
|
$array = $_POST;
|
|
$array['userid'] = userid();
|
|
$userquery->change_email($array);
|
|
}
|
|
|
|
//Changing User Password
|
|
if(isset($_POST['change_password']))
|
|
{
|
|
$array = $_POST;
|
|
$array['userid'] = userid();
|
|
$userquery->change_password($array);
|
|
}
|
|
|
|
//Banning Users
|
|
if(isset($_POST['block_users']))
|
|
{
|
|
$userquery->block_users($_POST['users']);
|
|
}
|
|
|
|
if ( mysql_clean($_GET['mode']) == 'make_avatar' ) {
|
|
|
|
$pid = mysql_clean( $_GET['pid'] );
|
|
$pid = $cbphoto->decode_key( $pid );
|
|
$pid = substr( $pid, 12, 12 );
|
|
$photo = $cbphoto->get_photo( $pid );
|
|
|
|
if ( !$photo ) {
|
|
e( lang('photo_not_exist') );
|
|
cb_show_page( false );
|
|
} else {
|
|
assign( 'photo', $photo );
|
|
if ( isset($_GET['set_avatar']) ) {
|
|
/* Run set avatar code */
|
|
$uid = userid();
|
|
$db->update( tbl('users'), array('avatar'), array( $uid.'_'.$photo['filename'].'.'.$photo['ext'] ), " userid = '".$uid."' " );
|
|
|
|
// redirect back to photo
|
|
redirect_to( $cbphoto->photo_links( $photo, 'view_photo' ) );
|
|
} else if( isset( $_POST['set_avatar']) ) {
|
|
/* Run make avatar code */
|
|
make_new_avatar();
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
$mode = $_GET['mode'];
|
|
|
|
|
|
|
|
switch($mode)
|
|
{
|
|
case 'account':
|
|
{
|
|
assign('on','account');
|
|
assign('mode','profile_settings');
|
|
}
|
|
break;
|
|
case 'profile':
|
|
{
|
|
assign('on','profile');
|
|
assign('mode','profile_settings');
|
|
}
|
|
break;
|
|
|
|
case 'avatar_bg':
|
|
{
|
|
assign('mode','avatar_bg');
|
|
}
|
|
break;
|
|
|
|
case 'make_avatar': {
|
|
assign('mode', 'make_avatar');
|
|
}
|
|
break;
|
|
|
|
case 'change_email':
|
|
{
|
|
assign('mode','change_email');
|
|
}
|
|
break;
|
|
|
|
case 'change_password':
|
|
{
|
|
assign('mode','change_password');
|
|
}
|
|
break;
|
|
|
|
case 'block_users':
|
|
{
|
|
assign('mode','block_users');
|
|
}
|
|
break;
|
|
|
|
case 'subscriptions':
|
|
{
|
|
//Removing subscription
|
|
if(isset($_GET['delete_subs']))
|
|
{
|
|
$sid = mysql_clean($_GET['delete_subs']);
|
|
$userquery->unsubscribe_user($sid);
|
|
}
|
|
assign('mode','subs');
|
|
assign('subs',$userquery->get_user_subscriptions(userid()));
|
|
}
|
|
break;
|
|
|
|
default:
|
|
{
|
|
assign('on','account');
|
|
assign('mode','profile_settings');
|
|
}
|
|
}
|
|
|
|
|
|
$udetails = $userquery->get_user_details(userid());
|
|
$profile = $userquery->get_user_profile($udetails['userid']);
|
|
$user_profile = array_merge($udetails,$profile);
|
|
//pr($Cbucket->header_files);
|
|
assign('user',$udetails);
|
|
assign('p',$user_profile);
|
|
|
|
|
|
subtitle(lang("user_manage_my_account"));
|
|
template_files('edit_account.html');
|
|
display_it();
|
|
?>
|