mirror of
https://bitbucket.org/smil3y/kdelibs.git
synced 2025-02-23 18:32:49 +00:00
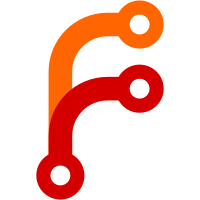
by using QLocale in KLocale and separating the date, time and numbers
conversion from translation KLocale actually gets extended locale
support from QLocale (which uses CLDR data v43 currently). translation
remains unaffected. for comparison here is the result of two function
calls the result of which should explain the whole change:
KLocale::allLanguagesList().size() = 669
KLocale::installedLanguages().size() = 68
the first number is locales Katie supports, the second being the
number of languages Katana is translated into
KSwitchLanguageDialog needs a rewrite but that is on the TODO
also copyrighting KCatalog to me because I rewrote it, for reference:
881b47b8ea
KCalendarSystem gets the middle finger - batteries not included for date
and time. extra calendar systems can, but are unlikely to be, supported
in the future
Signed-off-by: Ivailo Monev <xakepa10@gmail.com>
101 lines
3.6 KiB
C++
101 lines
3.6 KiB
C++
/* This file is part of the KDE libraries
|
|
Copyright (C) 2004, 2010 Joseph Wenninger <jowenn@kde.org>
|
|
|
|
This library is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU Library General Public
|
|
License version 2 as published by the Free Software Foundation.
|
|
|
|
This library is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
Library General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Library General Public License
|
|
along with this library; see the file COPYING.LIB. If not, write to
|
|
the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor,
|
|
Boston, MA 02110-1301, USA.
|
|
*/
|
|
|
|
#include "templateinterface.h"
|
|
#include "document.h"
|
|
#include "view.h"
|
|
|
|
#include <klocale.h>
|
|
#include <kglobal.h>
|
|
#include <kmessagebox.h>
|
|
#include <kemailsettings.h>
|
|
#include <kuser.h>
|
|
#include <kdebug.h>
|
|
|
|
#include <QString>
|
|
#include <QDateTime>
|
|
#include <QRegExp>
|
|
#include <QHostInfo>
|
|
|
|
#define DUMMY_VALUE "!KTE:TEMPLATEHANDLER_DUMMY_VALUE!"
|
|
|
|
using namespace KTextEditor;
|
|
|
|
bool TemplateInterface::insertTemplateText(const Cursor& insertPosition, const QString &templateString, const QMap<QString, QString> &initialValues)
|
|
{
|
|
// NOTE: THE IMPLEMENTATION WILL HANDLE %{cursor}
|
|
|
|
QDateTime datetime = QDateTime::currentDateTime();
|
|
QDate date = datetime.date();
|
|
QTime time = datetime.time();
|
|
QWidget *parentWindow = dynamic_cast<QWidget*>(this);
|
|
|
|
QMap<QString, QString> enhancedInitValues(initialValues);
|
|
if (templateString.contains(QLatin1String("%{loginname}"))) {
|
|
enhancedInitValues["loginname"] = KUser().loginName();
|
|
}
|
|
|
|
if (templateString.contains(QLatin1String("%{fullname}"))) {
|
|
KEMailSettings mailsettings;
|
|
const QString fullname = mailsettings.getSetting(KEMailSettings::RealName);
|
|
if (fullname.isEmpty()) {
|
|
KMessageBox::sorry(parentWindow,i18n("The template needs information about you but it is not available.\n The information can be set set from system settings."));
|
|
return false;
|
|
}
|
|
enhancedInitValues["fullname"] = fullname;
|
|
}
|
|
|
|
if (templateString.contains(QLatin1String("%{email}"))) {
|
|
KEMailSettings mailsettings;
|
|
const QString email = mailsettings.getSetting(KEMailSettings::EmailAddress);
|
|
if (email.isEmpty()) {
|
|
KMessageBox::sorry(parentWindow,i18n("The template needs information about you but it is not available.\n The information can be set set from system settings."));
|
|
return false;
|
|
}
|
|
enhancedInitValues["email"] = email;
|
|
}
|
|
|
|
if (templateString.contains(QLatin1String("%{date}"))) {
|
|
enhancedInitValues["date"] = KGlobal::locale()->formatDate(date, QLocale::ShortFormat);
|
|
}
|
|
|
|
if (templateString.contains(QLatin1String("%{time}"))) {
|
|
enhancedInitValues["time"] = KGlobal::locale()->formatTime(time, QLocale::ShortFormat);
|
|
}
|
|
|
|
const QLocale locale = KGlobal::locale()->toLocale();
|
|
if (templateString.contains(QLatin1String("%{year}"))) {
|
|
enhancedInitValues["year"] = locale.toString(date, "yyyy");
|
|
}
|
|
|
|
if (templateString.contains(QLatin1String("%{month}"))) {
|
|
enhancedInitValues["month"] = locale.toString(date, "MM");
|
|
}
|
|
|
|
if (templateString.contains(QLatin1String("%{day}"))) {
|
|
enhancedInitValues["day"] = locale.toString(date, "dd");
|
|
}
|
|
|
|
if (templateString.contains(QLatin1String("%{hostname}"))) {
|
|
enhancedInitValues["hostname"] = QHostInfo::localHostName();
|
|
}
|
|
|
|
return insertTemplateTextImplementation(insertPosition, templateString, enhancedInitValues);
|
|
}
|
|
|
|
// kate: space-indent on; indent-width 2; replace-tabs on;
|