mirror of
https://bitbucket.org/smil3y/kde-extraapps.git
synced 2025-02-24 19:02:53 +00:00
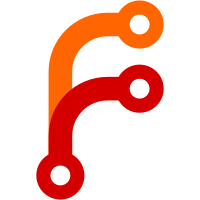
generally speaking trying to guess MPRIS interfaces is brute-force guess - it works kinda. the check that was used before this change however does not account for applications that are not categorized as audio or music player but do have MPRIS interface (chromium does). side note: tested only the MPRIS v2 interface Signed-off-by: Ivailo Monev <xakepa10@gmail.com>
95 lines
2.6 KiB
C++
95 lines
2.6 KiB
C++
/*
|
|
* Icon Task Manager
|
|
*
|
|
* Copyright 2011 Craig Drummond <craig@kde.org>
|
|
*
|
|
* ----
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; see the file COPYING. If not, write to
|
|
* the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor,
|
|
* Boston, MA 02110-1301, USA.
|
|
*/
|
|
|
|
#ifndef __MEDIABUTTONS_H__
|
|
#define __MEDIABUTTONS_H__
|
|
|
|
#include <QtCore/QObject>
|
|
#include <QtCore/QMap>
|
|
#include <QSet>
|
|
|
|
#include <QDBusServiceWatcher>
|
|
class OrgMprisMediaPlayer2PlayerInterface;
|
|
class OrgFreedesktopMediaPlayerInterface;
|
|
|
|
class MediaButtons : public QObject
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
class Interface
|
|
{
|
|
public:
|
|
Interface(OrgFreedesktopMediaPlayerInterface *o) : v1(o), v2(0) { }
|
|
Interface(OrgMprisMediaPlayer2PlayerInterface *t) : v1(0), v2(t) { }
|
|
~Interface();
|
|
|
|
bool isV1() const {
|
|
return 0 != v1;
|
|
}
|
|
bool isV2() const {
|
|
return 0 != v2;
|
|
}
|
|
|
|
void next();
|
|
void previous();
|
|
void playPause();
|
|
QString playbackStatus();
|
|
QString service();
|
|
private:
|
|
OrgFreedesktopMediaPlayerInterface *v1;
|
|
OrgMprisMediaPlayer2PlayerInterface *v2;
|
|
};
|
|
|
|
static MediaButtons * self();
|
|
|
|
MediaButtons();
|
|
|
|
void setEnabled(bool en);
|
|
bool isEnabled() const {
|
|
return m_enabled;
|
|
}
|
|
bool isMediaApp(const QString &desktopEntry);
|
|
void next(const QString &name, int pid = 0);
|
|
void previous(const QString &name, int pid = 0);
|
|
void playPause(const QString &name, int pid = 0);
|
|
QString playbackStatus(const QString &name, int pid = 0);
|
|
|
|
private Q_SLOTS:
|
|
void serviceOwnerChanged(const QString &name, const QString &oldOwner, const QString &newOwner);
|
|
|
|
private:
|
|
void readConfig();
|
|
Interface * getInterface(const QString &name, int pid);
|
|
Interface * getV2Interface(const QString &name);
|
|
Interface * getV1Interface(const QString &name);
|
|
|
|
private:
|
|
QDBusServiceWatcher *m_watcher;
|
|
QMap<QString, Interface *> m_interfaces;
|
|
QMap<QString, QString> m_aliases;
|
|
QSet<QString> m_ignore;
|
|
bool m_enabled;
|
|
};
|
|
|
|
#endif
|