mirror of
https://github.com/u-boot/u-boot.git
synced 2025-04-20 20:04:46 +00:00
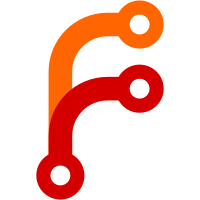
Previously values greater than 255 were implicitly truncated. Add some stricter checking to reject addresses with components >255. With the input "1234192.168.1.1" the old behaviour would truncate the address to 192.168.1.1. New behaviour rejects the string outright and returns 0.0.0.0, which for the purposes of IP addresses can be considered an error. Signed-off-by: Chris Packham <judge.packham@gmail.com>
40 lines
756 B
C
40 lines
756 B
C
/*
|
|
* Generic network code. Moved from net.c
|
|
*
|
|
* Copyright 1994 - 2000 Neil Russell.
|
|
* Copyright 2000 Roland Borde
|
|
* Copyright 2000 Paolo Scaffardi
|
|
* Copyright 2000-2002 Wolfgang Denk, wd@denx.de
|
|
* Copyright 2009 Dirk Behme, dirk.behme@googlemail.com
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
|
|
struct in_addr string_to_ip(const char *s)
|
|
{
|
|
struct in_addr addr;
|
|
char *e;
|
|
int i;
|
|
|
|
addr.s_addr = 0;
|
|
if (s == NULL)
|
|
return addr;
|
|
|
|
for (addr.s_addr = 0, i = 0; i < 4; ++i) {
|
|
ulong val = s ? simple_strtoul(s, &e, 10) : 0;
|
|
if (val > 255) {
|
|
addr.s_addr = 0;
|
|
return addr;
|
|
}
|
|
addr.s_addr <<= 8;
|
|
addr.s_addr |= (val & 0xFF);
|
|
if (s) {
|
|
s = (*e) ? e+1 : e;
|
|
}
|
|
}
|
|
|
|
addr.s_addr = htonl(addr.s_addr);
|
|
return addr;
|
|
}
|