mirror of
https://github.com/u-boot/u-boot.git
synced 2025-04-18 10:54:37 +00:00
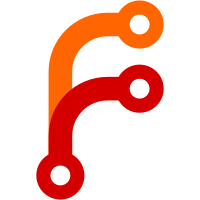
Perform all the block device creation only once, after the driver itself successfully bound. Do not do this in uclass post bind, as this might be triggered multiple times. For example the ut_dm_host test triggers this and triggers a memory leak that way, since there are now multiple block devices created using the blk_create_devicef() . To retain the old probe-on-boot behavior, set DM_FLAG_PROBE_AFTER_BIND flag in uclass post_bind callback, so the driver model would probe the driver at the right time. Rename the function as well, to match similar functions in other block-related subsystems, like the mmc one. Signed-off-by: Marek Vasut <marek.vasut+renesas@mailbox.org>
70 lines
1.6 KiB
C
70 lines
1.6 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Copyright 2023 Arm Limited and/or its affiliates <open-source-office@arm.com>
|
|
*
|
|
* Authors:
|
|
* Abdellatif El Khlifi <abdellatif.elkhlifi@arm.com>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <dm.h>
|
|
#include <log.h>
|
|
#if CONFIG_IS_ENABLED(SANDBOX64)
|
|
#include <asm/test.h>
|
|
#endif
|
|
#include <linux/bitops.h>
|
|
#include "nvmxip.h"
|
|
|
|
/* LBA Macros */
|
|
|
|
#define DEFAULT_LBA_SHIFT 10 /* 1024 bytes per block */
|
|
#define DEFAULT_LBA_COUNT 1024 /* block count */
|
|
|
|
#define DEFAULT_LBA_SZ BIT(DEFAULT_LBA_SHIFT)
|
|
|
|
int nvmxip_probe(struct udevice *udev)
|
|
{
|
|
int ret;
|
|
struct udevice *bdev = NULL;
|
|
char bdev_name[NVMXIP_BLKDEV_NAME_SZ + 1];
|
|
int devnum;
|
|
|
|
#if CONFIG_IS_ENABLED(SANDBOX64)
|
|
sandbox_set_enable_memio(true);
|
|
#endif
|
|
|
|
devnum = uclass_id_count(UCLASS_NVMXIP);
|
|
snprintf(bdev_name, NVMXIP_BLKDEV_NAME_SZ, "blk#%d", devnum);
|
|
|
|
ret = blk_create_devicef(udev, NVMXIP_BLKDRV_NAME, bdev_name, UCLASS_NVMXIP,
|
|
devnum, DEFAULT_LBA_SZ,
|
|
DEFAULT_LBA_COUNT, &bdev);
|
|
if (ret) {
|
|
log_err("[%s]: failure during creation of the block device %s, error %d\n",
|
|
udev->name, bdev_name, ret);
|
|
return ret;
|
|
}
|
|
|
|
ret = blk_probe_or_unbind(bdev);
|
|
if (ret) {
|
|
log_err("[%s]: failure during probing the block device %s, error %d\n",
|
|
udev->name, bdev_name, ret);
|
|
return ret;
|
|
}
|
|
|
|
log_info("[%s]: the block device %s ready for use\n", udev->name, bdev_name);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int nvmxip_post_bind(struct udevice *udev)
|
|
{
|
|
dev_or_flags(udev, DM_FLAG_PROBE_AFTER_BIND);
|
|
return 0;
|
|
}
|
|
|
|
UCLASS_DRIVER(nvmxip) = {
|
|
.name = "nvmxip",
|
|
.id = UCLASS_NVMXIP,
|
|
.post_bind = nvmxip_post_bind,
|
|
};
|