mirror of
https://github.com/u-boot/u-boot.git
synced 2025-04-24 22:36:05 +00:00
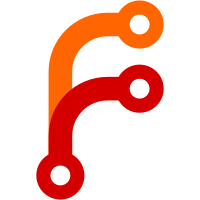
This fixture name is quite long and results in lots of verbose code. We know this is U-Boot so the 'u_boot_' part is not necessary. But it is also a bit of a misnomer, since it provides access to all the information available to tests. It is not just the console. It would be too confusing to use con as it would be confused with config and it is probably too short. So shorten it to 'ubman'. Signed-off-by: Simon Glass <sjg@chromium.org> Link: https://lore.kernel.org/u-boot/CAFLszTgPa4aT_J9h9pqeTtLCVn4x2JvLWRcWRD8NaN3uoSAtyA@mail.gmail.com/
98 lines
3.3 KiB
Python
98 lines
3.3 KiB
Python
# SPDX-License-Identifier: GPL-2.0
|
|
# (C) Copyright 2018 Texas Instruments, <www.ti.com>
|
|
|
|
# Test A/B update commands.
|
|
|
|
import os
|
|
import pytest
|
|
import u_boot_utils
|
|
|
|
class ABTestDiskImage(object):
|
|
"""Disk Image used by the A/B tests."""
|
|
|
|
def __init__(self, ubman):
|
|
"""Initialize a new ABTestDiskImage object.
|
|
|
|
Args:
|
|
ubman: A U-Boot console.
|
|
|
|
Returns:
|
|
Nothing.
|
|
"""
|
|
|
|
filename = 'test_ab_disk_image.bin'
|
|
|
|
persistent = ubman.config.persistent_data_dir + '/' + filename
|
|
self.path = ubman.config.result_dir + '/' + filename
|
|
|
|
with u_boot_utils.persistent_file_helper(ubman.log, persistent):
|
|
if os.path.exists(persistent):
|
|
ubman.log.action('Disk image file ' + persistent +
|
|
' already exists')
|
|
else:
|
|
ubman.log.action('Generating ' + persistent)
|
|
fd = os.open(persistent, os.O_RDWR | os.O_CREAT)
|
|
os.ftruncate(fd, 524288)
|
|
os.close(fd)
|
|
cmd = ('sgdisk', persistent)
|
|
u_boot_utils.run_and_log(ubman, cmd)
|
|
|
|
cmd = ('sgdisk', '--new=1:64:512', '--change-name=1:misc',
|
|
persistent)
|
|
u_boot_utils.run_and_log(ubman, cmd)
|
|
cmd = ('sgdisk', '--load-backup=' + persistent)
|
|
u_boot_utils.run_and_log(ubman, cmd)
|
|
|
|
cmd = ('cp', persistent, self.path)
|
|
u_boot_utils.run_and_log(ubman, cmd)
|
|
|
|
di = None
|
|
@pytest.fixture(scope='function')
|
|
def ab_disk_image(ubman):
|
|
global di
|
|
if not di:
|
|
di = ABTestDiskImage(ubman)
|
|
return di
|
|
|
|
def ab_dump(ubman, slot_num, crc):
|
|
output = ubman.run_command('bcb ab_dump host 0#misc')
|
|
header, slot0, slot1 = output.split('\r\r\n\r\r\n')
|
|
slots = [slot0, slot1]
|
|
slot_suffixes = ['_a', '_b']
|
|
|
|
header = dict(map(lambda x: map(str.strip, x.split(':')), header.split('\r\r\n')))
|
|
assert header['Bootloader Control'] == '[misc]'
|
|
assert header['Active Slot'] == slot_suffixes[slot_num]
|
|
assert header['Magic Number'] == '0x42414342'
|
|
assert header['Version'] == '1'
|
|
assert header['Number of Slots'] == '2'
|
|
assert header['Recovery Tries Remaining'] == '0'
|
|
assert header['CRC'] == '{} (Valid)'.format(crc)
|
|
|
|
slot = dict(map(lambda x: map(str.strip, x.split(':')), slots[slot_num].split('\r\r\n\t- ')[1:]))
|
|
assert slot['Priority'] == '15'
|
|
assert slot['Tries Remaining'] == '6'
|
|
assert slot['Successful Boot'] == '0'
|
|
assert slot['Verity Corrupted'] == '0'
|
|
|
|
@pytest.mark.boardspec('sandbox')
|
|
@pytest.mark.buildconfigspec('android_ab')
|
|
@pytest.mark.buildconfigspec('cmd_bcb')
|
|
@pytest.mark.requiredtool('sgdisk')
|
|
def test_ab(ab_disk_image, ubman):
|
|
"""Test the 'bcb ab_select' command."""
|
|
|
|
ubman.run_command('host bind 0 ' + ab_disk_image.path)
|
|
|
|
output = ubman.run_command('bcb ab_select slot_name host 0#misc')
|
|
assert 're-initializing A/B metadata' in output
|
|
assert 'Attempting slot a, tries remaining 7' in output
|
|
output = ubman.run_command('printenv slot_name')
|
|
assert 'slot_name=a' in output
|
|
ab_dump(ubman, 0, '0xd438d1b9')
|
|
|
|
output = ubman.run_command('bcb ab_select slot_name host 0:1')
|
|
assert 'Attempting slot b, tries remaining 7' in output
|
|
output = ubman.run_command('printenv slot_name')
|
|
assert 'slot_name=b' in output
|
|
ab_dump(ubman, 1, '0x011ec016')
|