mirror of
https://github.com/u-boot/u-boot.git
synced 2025-04-16 01:44:34 +00:00
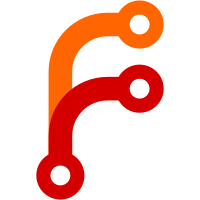
This fixture name is quite long and results in lots of verbose code. We know this is U-Boot so the 'u_boot_' part is not necessary. But it is also a bit of a misnomer, since it provides access to all the information available to tests. It is not just the console. It would be too confusing to use con as it would be confused with config and it is probably too short. So shorten it to 'ubman'. Signed-off-by: Simon Glass <sjg@chromium.org> Link: https://lore.kernel.org/u-boot/CAFLszTgPa4aT_J9h9pqeTtLCVn4x2JvLWRcWRD8NaN3uoSAtyA@mail.gmail.com/
45 lines
1.5 KiB
Python
45 lines
1.5 KiB
Python
# SPDX-License-Identifier: GPL-2.0
|
|
# Copyright (c) 2015-2016, NVIDIA CORPORATION. All rights reserved.
|
|
|
|
# Test basic shell functionality, such as commands separate by semi-colons.
|
|
|
|
import pytest
|
|
|
|
pytestmark = pytest.mark.buildconfigspec('cmd_echo')
|
|
|
|
def test_shell_execute(ubman):
|
|
"""Test any shell command."""
|
|
|
|
response = ubman.run_command('echo hello')
|
|
assert response.strip() == 'hello'
|
|
|
|
def test_shell_semicolon_two(ubman):
|
|
"""Test two shell commands separate by a semi-colon."""
|
|
|
|
cmd = 'echo hello; echo world'
|
|
response = ubman.run_command(cmd)
|
|
# This validation method ignores the exact whitespace between the strings
|
|
assert response.index('hello') < response.index('world')
|
|
|
|
def test_shell_semicolon_three(ubman):
|
|
"""Test three shell commands separate by a semi-colon, with variable
|
|
expansion dependencies between them."""
|
|
|
|
cmd = 'setenv list 1; setenv list ${list}2; setenv list ${list}3; ' + \
|
|
'echo ${list}'
|
|
response = ubman.run_command(cmd)
|
|
assert response.strip() == '123'
|
|
ubman.run_command('setenv list')
|
|
|
|
def test_shell_run(ubman):
|
|
"""Test the "run" shell command."""
|
|
|
|
ubman.run_command('setenv foo \'setenv monty 1; setenv python 2\'')
|
|
ubman.run_command('run foo')
|
|
response = ubman.run_command('echo ${monty}')
|
|
assert response.strip() == '1'
|
|
response = ubman.run_command('echo ${python}')
|
|
assert response.strip() == '2'
|
|
ubman.run_command('setenv foo')
|
|
ubman.run_command('setenv monty')
|
|
ubman.run_command('setenv python')
|