mirror of
https://github.com/u-boot/u-boot.git
synced 2025-04-11 07:24:46 +00:00
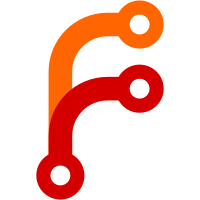
The function sha256_csum_wd is defined in lib/sha256.c and in lib/mbedtls/sha256.c. To avoid duplicating this function (and future function), we move this function to the file lib/sha256_common.c Reviewed-by: Raymond Mao <raymond.mao@linaro.org> Signed-off-by: Philippe Reynes <philippe.reynes@softathome.com>
50 lines
1.1 KiB
C
50 lines
1.1 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* FIPS-180-2 compliant SHA-256 implementation
|
|
*
|
|
* Copyright (C) 2001-2003 Christophe Devine
|
|
*/
|
|
|
|
#ifndef USE_HOSTCC
|
|
#include <u-boot/schedule.h>
|
|
#endif /* USE_HOSTCC */
|
|
#include <string.h>
|
|
#include <u-boot/sha256.h>
|
|
|
|
#include <linux/compiler_attributes.h>
|
|
|
|
/*
|
|
* Output = SHA-256( input buffer ). Trigger the watchdog every 'chunk_sz'
|
|
* bytes of input processed.
|
|
*/
|
|
void sha256_csum_wd(const unsigned char *input, unsigned int ilen,
|
|
unsigned char *output, unsigned int chunk_sz)
|
|
{
|
|
sha256_context ctx;
|
|
#if !defined(USE_HOSTCC) && \
|
|
(defined(CONFIG_HW_WATCHDOG) || defined(CONFIG_WATCHDOG))
|
|
const unsigned char *end;
|
|
unsigned char *curr;
|
|
int chunk;
|
|
#endif
|
|
|
|
sha256_starts(&ctx);
|
|
|
|
#if !defined(USE_HOSTCC) && \
|
|
(defined(CONFIG_HW_WATCHDOG) || defined(CONFIG_WATCHDOG))
|
|
curr = (unsigned char *)input;
|
|
end = input + ilen;
|
|
while (curr < end) {
|
|
chunk = end - curr;
|
|
if (chunk > chunk_sz)
|
|
chunk = chunk_sz;
|
|
sha256_update(&ctx, curr, chunk);
|
|
curr += chunk;
|
|
schedule();
|
|
}
|
|
#else
|
|
sha256_update(&ctx, input, ilen);
|
|
#endif
|
|
|
|
sha256_finish(&ctx, output);
|
|
}
|