mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-17 18:14:24 +00:00
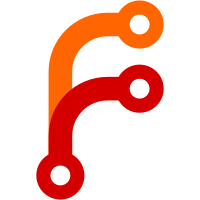
In order to ease the introduction of a new BSEC3 driver for STM32MP25, the BSEC2 driver is reworked. Unused functions are removed. The bsec_base global variable is removed in favor of the macro BSEC_BASE. A rework is also done around function checking the state of BSEC. Change-Id: I1ad76cb67333ab9a8fa1d65db34d74a712bf1190 Signed-off-by: Patrick Delaunay <patrick.delaunay@foss.st.com> Signed-off-by: Yann Gautier <yann.gautier@st.com>
55 lines
1.1 KiB
C
55 lines
1.1 KiB
C
/*
|
|
* Copyright (c) 2016-2024, STMicroelectronics - All Rights Reserved
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
|
|
|
|
#include <common/debug.h>
|
|
#include <drivers/st/bsec.h>
|
|
#include <drivers/st/bsec2_reg.h>
|
|
|
|
#include <platform_def.h>
|
|
#include <stm32mp1_smc.h>
|
|
|
|
#include "bsec_svc.h"
|
|
|
|
uint32_t bsec_main(uint32_t x1, uint32_t x2, uint32_t x3,
|
|
uint32_t *ret_otp_value)
|
|
{
|
|
uint32_t result;
|
|
uint32_t tmp_data = 0U;
|
|
|
|
switch (x1) {
|
|
case STM32_SMC_READ_SHADOW:
|
|
result = bsec_read_otp(ret_otp_value, x2);
|
|
break;
|
|
case STM32_SMC_PROG_OTP:
|
|
*ret_otp_value = 0U;
|
|
result = bsec_program_otp(x3, x2);
|
|
break;
|
|
case STM32_SMC_WRITE_SHADOW:
|
|
*ret_otp_value = 0U;
|
|
result = bsec_write_otp(x3, x2);
|
|
break;
|
|
case STM32_SMC_READ_OTP:
|
|
*ret_otp_value = 0U;
|
|
result = bsec_read_otp(&tmp_data, x2);
|
|
if (result != BSEC_OK) {
|
|
break;
|
|
}
|
|
|
|
result = bsec_shadow_read_otp(ret_otp_value, x2);
|
|
if (result != BSEC_OK) {
|
|
break;
|
|
}
|
|
|
|
result = bsec_write_otp(tmp_data, x2);
|
|
break;
|
|
|
|
default:
|
|
return STM32_SMC_INVALID_PARAMS;
|
|
}
|
|
|
|
return (result == BSEC_OK) ? STM32_SMC_OK : STM32_SMC_FAILED;
|
|
}
|