mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-16 01:24:27 +00:00
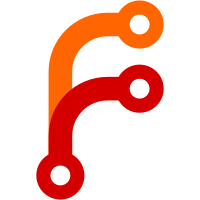
The framework currently supports QE feature only for Macronix devices. Kioxia devices also support this feature, but this feature can not be set based on the manufacturer ID as Kioxia first SPI NAND generation does not support the QE feature when the second generation does. Use a flag to manage QE feature. This flag will be added at board level to manage the device. Change-Id: I7a3683a2df8739967b17b4abbec32c51bf206b93 Signed-off-by: Christophe Kerello <christophe.kerello@foss.st.com>
53 lines
1.4 KiB
C
53 lines
1.4 KiB
C
/*
|
|
* Copyright (c) 2019-2023, STMicroelectronics - All Rights Reserved
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
|
|
#ifndef DRIVERS_SPI_NAND_H
|
|
#define DRIVERS_SPI_NAND_H
|
|
|
|
#include <drivers/nand.h>
|
|
#include <drivers/spi_mem.h>
|
|
|
|
#define SPI_NAND_OP_GET_FEATURE 0x0FU
|
|
#define SPI_NAND_OP_SET_FEATURE 0x1FU
|
|
#define SPI_NAND_OP_READ_ID 0x9FU
|
|
#define SPI_NAND_OP_LOAD_PAGE 0x13U
|
|
#define SPI_NAND_OP_RESET 0xFFU
|
|
#define SPI_NAND_OP_READ_FROM_CACHE 0x03U
|
|
#define SPI_NAND_OP_READ_FROM_CACHE_2X 0x3BU
|
|
#define SPI_NAND_OP_READ_FROM_CACHE_4X 0x6BU
|
|
|
|
/* Configuration register */
|
|
#define SPI_NAND_REG_CFG 0xB0U
|
|
#define SPI_NAND_CFG_ECC_EN BIT(4)
|
|
#define SPI_NAND_CFG_QE BIT(0)
|
|
|
|
/* Status register */
|
|
#define SPI_NAND_REG_STATUS 0xC0U
|
|
#define SPI_NAND_STATUS_BUSY BIT(0)
|
|
#define SPI_NAND_STATUS_ECC_UNCOR BIT(5)
|
|
|
|
/* Flags for specific configuration */
|
|
#define SPI_NAND_HAS_QE_BIT BIT(0)
|
|
|
|
struct spinand_device {
|
|
struct nand_device *nand_dev;
|
|
struct spi_mem_op spi_read_cache_op;
|
|
uint32_t flags;
|
|
uint8_t cfg_cache; /* Cached value of SPI NAND device register CFG */
|
|
};
|
|
|
|
int spi_nand_init(unsigned long long *size, unsigned int *erase_size);
|
|
|
|
/*
|
|
* Platform can implement this to override default SPI-NAND instance
|
|
* configuration.
|
|
*
|
|
* @device: target SPI-NAND instance.
|
|
* Return 0 on success, negative value otherwise.
|
|
*/
|
|
int plat_get_spi_nand_data(struct spinand_device *device);
|
|
|
|
#endif /* DRIVERS_SPI_NAND_H */
|