mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-18 10:34:19 +00:00
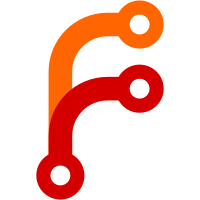
The S32CC is an umbrella for S32G2, S32G3 and S32R45 SoCs; therefore, this clock driver will be used for all of these families. Change-Id: Iede5371b212b67cf494a033c62fbfdcbe9b1a879 Signed-off-by: Ghennadi Procopciuc <ghennadi.procopciuc@nxp.com>
59 lines
1 KiB
C
59 lines
1 KiB
C
/*
|
|
* Copyright 2024 NXP
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
#include <errno.h>
|
|
|
|
#include <drivers/clk.h>
|
|
|
|
static int s32cc_clk_enable(unsigned long id)
|
|
{
|
|
return -ENOTSUP;
|
|
}
|
|
|
|
static void s32cc_clk_disable(unsigned long id)
|
|
{
|
|
}
|
|
|
|
static bool s32cc_clk_is_enabled(unsigned long id)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
static unsigned long s32cc_clk_get_rate(unsigned long id)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static int s32cc_clk_set_rate(unsigned long id, unsigned long rate,
|
|
unsigned long *orate)
|
|
{
|
|
return -ENOTSUP;
|
|
}
|
|
|
|
static int s32cc_clk_get_parent(unsigned long id)
|
|
{
|
|
return -ENOTSUP;
|
|
}
|
|
|
|
static int s32cc_clk_set_parent(unsigned long id, unsigned long parent_id)
|
|
{
|
|
return -ENOTSUP;
|
|
}
|
|
|
|
void s32cc_clk_register_drv(void)
|
|
{
|
|
static const struct clk_ops s32cc_clk_ops = {
|
|
.enable = s32cc_clk_enable,
|
|
.disable = s32cc_clk_disable,
|
|
.is_enabled = s32cc_clk_is_enabled,
|
|
.get_rate = s32cc_clk_get_rate,
|
|
.set_rate = s32cc_clk_set_rate,
|
|
.get_parent = s32cc_clk_get_parent,
|
|
.set_parent = s32cc_clk_set_parent,
|
|
};
|
|
|
|
clk_register(&s32cc_clk_ops);
|
|
}
|
|
|