mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-17 10:04:26 +00:00
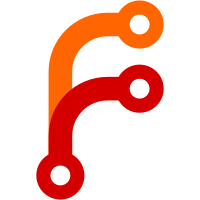
Just like for SPE, we need to synchronize TRBE samples before we change the context to ensure everything goes where it was intended to. If that is not done, the in-flight entries might use any piece of now incorrect context as there are no implicit ordering requirements. Prior to root context, the buffer drain hooks would have done that. But now that must happen much earlier. So add a tsb to prepare_el3_entry as well. Annoyingly, the barrier can be reordered relative to other instructions by default (rule RCKVWP). So add an isb after the psb/tsb to assure that they are ordered, at least as far as context is concerned. Then, drop the buffer draining hooks. Everything they need to do is already done by now. There's a notable difference in that there are no dsb-s now. Since EL3 does not access the buffers or the feature specific context, we don't need to wait for them to finish. Finally, drop a stray isb in the context saving macro. It is now absorbed into root context, but was missed. Change-Id: I30797a40ac7f91d0bb71ad271a1597e85092ccd5 Signed-off-by: Boyan Karatotev <boyan.karatotev@arm.com>
60 lines
1.7 KiB
C
60 lines
1.7 KiB
C
/*
|
|
* Copyright (c) 2021-2024, Arm Limited. All rights reserved.
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
|
|
#include <arch.h>
|
|
#include <arch_features.h>
|
|
#include <arch_helpers.h>
|
|
#include <lib/extensions/trbe.h>
|
|
|
|
void trbe_enable(cpu_context_t *ctx)
|
|
{
|
|
el3_state_t *state = get_el3state_ctx(ctx);
|
|
u_register_t mdcr_el3_val = read_ctx_reg(state, CTX_MDCR_EL3);
|
|
|
|
/*
|
|
* MDCR_EL3.NSTBE = 0b0
|
|
* Trace Buffer owning Security state is Non-secure state. If FEAT_RME
|
|
* is not implemented, this field is RES0.
|
|
*
|
|
* MDCR_EL3.NSTB = 0b11
|
|
* Allow access of trace buffer control registers from NS-EL1 and
|
|
* NS-EL2, tracing is prohibited in Secure and Realm state (if
|
|
* implemented).
|
|
*/
|
|
mdcr_el3_val |= MDCR_NSTB(MDCR_NSTB_EL1);
|
|
mdcr_el3_val &= ~(MDCR_NSTBE_BIT);
|
|
write_ctx_reg(state, CTX_MDCR_EL3, mdcr_el3_val);
|
|
}
|
|
|
|
void trbe_disable(cpu_context_t *ctx)
|
|
{
|
|
el3_state_t *state = get_el3state_ctx(ctx);
|
|
u_register_t mdcr_el3_val = read_ctx_reg(state, CTX_MDCR_EL3);
|
|
|
|
/*
|
|
* MDCR_EL3.NSTBE = 0b0
|
|
* Trace Buffer owning Security state is secure state. If FEAT_RME
|
|
* is not implemented, this field is RES0.
|
|
*
|
|
* MDCR_EL3.NSTB = 0b00
|
|
* Clear these bits to disable access of trace buffer control registers
|
|
* from lower ELs in any security state.
|
|
*/
|
|
mdcr_el3_val &= ~(MDCR_NSTB(MDCR_NSTB_EL1));
|
|
mdcr_el3_val &= ~(MDCR_NSTBE_BIT);
|
|
write_ctx_reg(state, CTX_MDCR_EL3, mdcr_el3_val);
|
|
}
|
|
|
|
void trbe_init_el2_unused(void)
|
|
{
|
|
/*
|
|
* MDCR_EL2.E2TB: Set to zero so that the trace Buffer
|
|
* owning exception level is NS-EL1 and, tracing is
|
|
* prohibited at NS-EL2. These bits are RES0 when
|
|
* FEAT_TRBE is not implemented.
|
|
*/
|
|
write_mdcr_el2(read_mdcr_el2() & ~MDCR_EL2_E2TB(MDCR_EL2_E2TB_EL1));
|
|
}
|