mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-07 21:33:54 +00:00
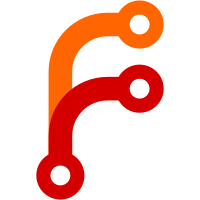
The current code is incredibly resilient to updates to the spec and has worked quite well so far. However, recent implementations expose a weakness in that this is rather slow. A large part of it is written in assembly, making it opaque to the compiler for optimisations. The future proofness requires reading registers that are effectively `volatile`, making it even harder for the compiler, as well as adding lots of implicit barriers, making it hard for the microarchitecutre to optimise as well. We can make a few assumptions, checked by a few well placed asserts, and remove a lot of this burden. For a start, at the moment there are 4 group 0 counters with static assignments. Contexting them is a trivial affair that doesn't need a loop. Similarly, there can only be up to 16 group 1 counters. Contexting them is a bit harder, but we can do with a single branch with a falling through switch. If/when both of these change, we have a pair of asserts and the feature detection mechanism to guard us against pretending that we support something we don't. We can drop contexting of the offset registers. They are fully accessible by EL2 and as such are its responsibility to preserve on powerdown. Another small thing we can do, is pass the core_pos into the hook. The caller already knows which core we're running on, we don't need to call this non-trivial function again. Finally, knowing this, we don't really need the auxiliary AMUs to be described by the device tree. Linux doesn't care at the moment, and any information we need for EL3 can be neatly placed in a simple array. All of this, combined with lifting the actual saving out of assembly, reduces the instructions to save the context from 180 to 40, including a lot fewer branches. The code is also much shorter and easier to read. Also propagate to aarch32 so that the two don't diverge too much. Change-Id: Ib62e6e9ba5be7fb9fb8965c8eee148d5598a5361 Signed-off-by: Boyan Karatotev <boyan.karatotev@arm.com>
646 lines
14 KiB
Text
646 lines
14 KiB
Text
/*
|
|
* Copyright (c) 2020-2024, Arm Limited. All rights reserved.
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
|
|
/* If SCMI power domain control is enabled */
|
|
#if TC_SCMI_PD_CTRL_EN
|
|
#define GPU_SCMI_PD_IDX (PLAT_MAX_CPUS_PER_CLUSTER + 1)
|
|
#define DPU_SCMI_PD_IDX (PLAT_MAX_CPUS_PER_CLUSTER + 2)
|
|
#endif /* TC_SCMI_PD_CTRL_EN */
|
|
|
|
/* Use SCMI controlled clocks */
|
|
#if TC_DPU_USE_SCMI_CLK
|
|
#define DPU_CLK_ATTR1 \
|
|
clocks = <&scmi_clk 0>; \
|
|
clock-names = "aclk"
|
|
|
|
#define DPU_CLK_ATTR2 \
|
|
clocks = <&scmi_clk 1>; \
|
|
clock-names = "pxclk"
|
|
|
|
#define DPU_CLK_ATTR3 \
|
|
clocks = <&scmi_clk 2>; \
|
|
clock-names = "pxclk" \
|
|
/* Use fixed clocks */
|
|
#else /* !TC_DPU_USE_SCMI_CLK */
|
|
#define DPU_CLK_ATTR1 \
|
|
clocks = <&dpu_aclk>; \
|
|
clock-names = "aclk"
|
|
|
|
#define DPU_CLK_ATTR2 \
|
|
clocks = <&dpu_pixel_clk>, <&dpu_aclk>; \
|
|
clock-names = "pxclk", "aclk"
|
|
|
|
#define DPU_CLK_ATTR3 DPU_CLK_ATTR2
|
|
#endif /* !TC_DPU_USE_SCMI_CLK */
|
|
|
|
/ {
|
|
compatible = "arm,tc";
|
|
interrupt-parent = <&gic>;
|
|
#address-cells = <2>;
|
|
#size-cells = <2>;
|
|
|
|
aliases {
|
|
serial0 = &os_uart;
|
|
};
|
|
|
|
cpus {
|
|
#address-cells = <1>;
|
|
#size-cells = <0>;
|
|
|
|
cpu-map {
|
|
cluster0 {
|
|
core0 {
|
|
cpu = <&CPU0>;
|
|
};
|
|
core1 {
|
|
cpu = <&CPU1>;
|
|
};
|
|
core2 {
|
|
cpu = <&CPU2>;
|
|
};
|
|
core3 {
|
|
cpu = <&CPU3>;
|
|
};
|
|
core4 {
|
|
cpu = <&CPU4>;
|
|
};
|
|
core5 {
|
|
cpu = <&CPU5>;
|
|
};
|
|
core6 {
|
|
cpu = <&CPU6>;
|
|
};
|
|
core7 {
|
|
cpu = <&CPU7>;
|
|
};
|
|
};
|
|
};
|
|
|
|
/*
|
|
* The timings below are just to demonstrate working cpuidle.
|
|
* These values may be inaccurate.
|
|
*/
|
|
idle-states {
|
|
entry-method = "psci";
|
|
|
|
CPU_SLEEP_0: cpu-sleep-0 {
|
|
compatible = "arm,idle-state";
|
|
arm,psci-suspend-param = <0x0010000>;
|
|
local-timer-stop;
|
|
entry-latency-us = <300>;
|
|
exit-latency-us = <1200>;
|
|
min-residency-us = <2000>;
|
|
};
|
|
CLUSTER_SLEEP_0: cluster-sleep-0 {
|
|
compatible = "arm,idle-state";
|
|
arm,psci-suspend-param = <0x1010000>;
|
|
local-timer-stop;
|
|
entry-latency-us = <400>;
|
|
exit-latency-us = <1200>;
|
|
min-residency-us = <2500>;
|
|
};
|
|
};
|
|
|
|
CPU0:cpu@0 {
|
|
device_type = "cpu";
|
|
compatible = "arm,armv8";
|
|
reg = <0x0>;
|
|
enable-method = "psci";
|
|
clocks = <&scmi_dvfs 0>;
|
|
cpu-idle-states = <&CPU_SLEEP_0 &CLUSTER_SLEEP_0>;
|
|
capacity-dmips-mhz = <LIT_CAPACITY>;
|
|
};
|
|
|
|
CPU1:cpu@100 {
|
|
device_type = "cpu";
|
|
compatible = "arm,armv8";
|
|
reg = <0x100>;
|
|
enable-method = "psci";
|
|
clocks = <&scmi_dvfs 0>;
|
|
cpu-idle-states = <&CPU_SLEEP_0 &CLUSTER_SLEEP_0>;
|
|
capacity-dmips-mhz = <LIT_CAPACITY>;
|
|
};
|
|
|
|
CPU2:cpu@200 {
|
|
device_type = "cpu";
|
|
compatible = "arm,armv8";
|
|
reg = <0x200>;
|
|
enable-method = "psci";
|
|
cpu-idle-states = <&CPU_SLEEP_0 &CLUSTER_SLEEP_0>;
|
|
};
|
|
|
|
CPU3:cpu@300 {
|
|
device_type = "cpu";
|
|
compatible = "arm,armv8";
|
|
reg = <0x300>;
|
|
enable-method = "psci";
|
|
cpu-idle-states = <&CPU_SLEEP_0 &CLUSTER_SLEEP_0>;
|
|
};
|
|
|
|
CPU4:cpu@400 {
|
|
device_type = "cpu";
|
|
compatible = "arm,armv8";
|
|
reg = <0x400>;
|
|
enable-method = "psci";
|
|
clocks = <&scmi_dvfs 1>;
|
|
cpu-idle-states = <&CPU_SLEEP_0 &CLUSTER_SLEEP_0>;
|
|
capacity-dmips-mhz = <MID_CAPACITY>;
|
|
};
|
|
|
|
CPU5:cpu@500 {
|
|
device_type = "cpu";
|
|
compatible = "arm,armv8";
|
|
reg = <0x500>;
|
|
enable-method = "psci";
|
|
clocks = <&scmi_dvfs 1>;
|
|
cpu-idle-states = <&CPU_SLEEP_0 &CLUSTER_SLEEP_0>;
|
|
capacity-dmips-mhz = <MID_CAPACITY>;
|
|
};
|
|
|
|
CPU6:cpu@600 {
|
|
device_type = "cpu";
|
|
compatible = "arm,armv8";
|
|
reg = <0x600>;
|
|
enable-method = "psci";
|
|
cpu-idle-states = <&CPU_SLEEP_0 &CLUSTER_SLEEP_0>;
|
|
};
|
|
|
|
CPU7:cpu@700 {
|
|
device_type = "cpu";
|
|
compatible = "arm,armv8";
|
|
reg = <0x700>;
|
|
enable-method = "psci";
|
|
cpu-idle-states = <&CPU_SLEEP_0 &CLUSTER_SLEEP_0>;
|
|
};
|
|
};
|
|
|
|
reserved-memory {
|
|
#address-cells = <2>;
|
|
#size-cells = <2>;
|
|
ranges;
|
|
|
|
linux,cma {
|
|
compatible = "shared-dma-pool";
|
|
reusable;
|
|
size = <0x0 0x8000000>;
|
|
linux,cma-default;
|
|
};
|
|
|
|
optee {
|
|
compatible = "restricted-dma-pool";
|
|
reg = <0x0 TC_NS_OPTEE_BASE 0x0 TC_NS_OPTEE_SIZE>;
|
|
};
|
|
|
|
};
|
|
|
|
memory {
|
|
device_type = "memory";
|
|
reg = <0x0 TC_NS_DRAM1_BASE 0x0 TC_NS_DRAM1_SIZE>,
|
|
<HI(PLAT_ARM_DRAM2_BASE) LO(PLAT_ARM_DRAM2_BASE)
|
|
HI(TC_NS_DRAM2_SIZE) LO(TC_NS_DRAM2_SIZE)>;
|
|
};
|
|
|
|
psci {
|
|
compatible = "arm,psci-1.0", "arm,psci-0.2";
|
|
method = "smc";
|
|
};
|
|
|
|
cpu-pmu-little {
|
|
compatible = LIT_CPU_PMU_COMPATIBLE;
|
|
interrupts = <GIC_PPI 7 IRQ_TYPE_LEVEL_HIGH &ppi_partition_little>;
|
|
status = "okay";
|
|
};
|
|
|
|
cpu-pmu-mid {
|
|
compatible = MID_CPU_PMU_COMPATIBLE;
|
|
interrupts = <GIC_PPI 7 IRQ_TYPE_LEVEL_HIGH &ppi_partition_mid>;
|
|
status = "okay";
|
|
};
|
|
|
|
cpu-pmu-big {
|
|
compatible = BIG_CPU_PMU_COMPATIBLE;
|
|
interrupts = <GIC_PPI 7 IRQ_TYPE_LEVEL_HIGH &ppi_partition_big>;
|
|
status = "okay";
|
|
};
|
|
|
|
sram: sram@6000000 {
|
|
compatible = "mmio-sram";
|
|
reg = <0x0 PLAT_ARM_NSRAM_BASE 0x0 PLAT_ARM_NSRAM_SIZE>;
|
|
|
|
#address-cells = <1>;
|
|
#size-cells = <1>;
|
|
ranges = <0 0x0 PLAT_ARM_NSRAM_BASE PLAT_ARM_NSRAM_SIZE>;
|
|
|
|
cpu_scp_scmi_a2p: scp-shmem@0 {
|
|
compatible = "arm,scmi-shmem";
|
|
reg = <0x0 0x80>;
|
|
};
|
|
};
|
|
|
|
mbox_db_rx: mhu@MHU_RX_ADDR {
|
|
compatible = MHU_RX_COMPAT;
|
|
reg = <0x0 ADDRESSIFY(MHU_RX_ADDR) 0x0 MHU_OFFSET>;
|
|
clocks = <&soc_refclk>;
|
|
clock-names = "apb_pclk";
|
|
#mbox-cells = <MHU_MBOX_CELLS>;
|
|
interrupts = <GIC_SPI MHU_RX_INT_NUM IRQ_TYPE_LEVEL_HIGH 0>;
|
|
interrupt-names = MHU_RX_INT_NAME;
|
|
};
|
|
|
|
mbox_db_tx: mhu@MHU_TX_ADDR {
|
|
compatible = MHU_TX_COMPAT;
|
|
reg = <0x0 ADDRESSIFY(MHU_TX_ADDR) 0x0 MHU_OFFSET>;
|
|
clocks = <&soc_refclk>;
|
|
clock-names = "apb_pclk";
|
|
#mbox-cells = <MHU_MBOX_CELLS>;
|
|
interrupt-names = MHU_TX_INT_NAME;
|
|
};
|
|
|
|
firmware {
|
|
scmi {
|
|
compatible = "arm,scmi";
|
|
mbox-names = "tx", "rx";
|
|
#address-cells = <1>;
|
|
#size-cells = <0>;
|
|
|
|
#if TC_SCMI_PD_CTRL_EN
|
|
scmi_devpd: protocol@11 {
|
|
reg = <0x11>;
|
|
#power-domain-cells = <1>;
|
|
};
|
|
#endif /* TC_SCMI_PD_CTRL_EN */
|
|
|
|
scmi_dvfs: protocol@13 {
|
|
reg = <0x13>;
|
|
#clock-cells = <1>;
|
|
};
|
|
|
|
scmi_clk: protocol@14 {
|
|
reg = <0x14>;
|
|
#clock-cells = <1>;
|
|
};
|
|
};
|
|
};
|
|
|
|
gic: interrupt-controller@GIC_CTRL_ADDR {
|
|
compatible = "arm,gic-v3";
|
|
#address-cells = <2>;
|
|
#interrupt-cells = <4>;
|
|
#size-cells = <2>;
|
|
ranges;
|
|
interrupt-controller;
|
|
reg = <0x0 0x30000000 0 0x10000>, /* GICD */
|
|
<0x0 0x30080000 0 GIC_GICR_OFFSET>; /* GICR */
|
|
interrupts = <GIC_PPI 0x9 IRQ_TYPE_LEVEL_LOW 0>;
|
|
};
|
|
|
|
timer {
|
|
compatible = "arm,armv8-timer";
|
|
interrupts = <GIC_PPI 13 IRQ_TYPE_LEVEL_LOW 0>,
|
|
<GIC_PPI 14 IRQ_TYPE_LEVEL_LOW 0>,
|
|
<GIC_PPI 11 IRQ_TYPE_LEVEL_LOW 0>,
|
|
<GIC_PPI 10 IRQ_TYPE_LEVEL_LOW 0>;
|
|
};
|
|
|
|
spe-pmu-mid {
|
|
compatible = "arm,statistical-profiling-extension-v1";
|
|
interrupts = <GIC_PPI 1 IRQ_TYPE_LEVEL_HIGH &ppi_partition_mid>;
|
|
status = "disabled";
|
|
};
|
|
|
|
spe-pmu-big {
|
|
compatible = "arm,statistical-profiling-extension-v1";
|
|
interrupts = <GIC_PPI 1 IRQ_TYPE_LEVEL_HIGH &ppi_partition_big>;
|
|
status = "disabled";
|
|
};
|
|
|
|
soc_refclk: refclk {
|
|
compatible = "fixed-clock";
|
|
#clock-cells = <0>;
|
|
clock-frequency = <1000000000>;
|
|
clock-output-names = "apb_pclk";
|
|
};
|
|
|
|
soc_refclk60mhz: refclk60mhz {
|
|
compatible = "fixed-clock";
|
|
#clock-cells = <0>;
|
|
clock-frequency = <60000000>;
|
|
clock-output-names = "iofpga_clk";
|
|
};
|
|
|
|
soc_uartclk: uartclk {
|
|
compatible = "fixed-clock";
|
|
#clock-cells = <0>;
|
|
clock-frequency = <TC_UARTCLK>;
|
|
clock-output-names = "uartclk";
|
|
};
|
|
|
|
/* soc_uart0 on FPGA, ap_ns_uart on FVP */
|
|
os_uart: serial@2a400000 {
|
|
compatible = "arm,pl011", "arm,primecell";
|
|
reg = <0x0 0x2A400000 0x0 UART_OFFSET>;
|
|
interrupts = <GIC_SPI 63 IRQ_TYPE_LEVEL_HIGH 0>;
|
|
clocks = <&soc_uartclk>, <&soc_refclk>;
|
|
clock-names = "uartclk", "apb_pclk";
|
|
status = "okay";
|
|
};
|
|
|
|
#if !TC_DPU_USE_SCMI_CLK
|
|
dpu_aclk: dpu_aclk {
|
|
compatible = "fixed-clock";
|
|
#clock-cells = <0>;
|
|
clock-frequency = <LCD_TIMING_CLK>;
|
|
clock-output-names = "fpga:dpu_aclk";
|
|
};
|
|
|
|
dpu_pixel_clk: dpu-pixel-clk {
|
|
compatible = "fixed-clock";
|
|
#clock-cells = <0>;
|
|
clock-frequency = <LCD_TIMING_CLK>;
|
|
clock-output-names = "pxclk";
|
|
};
|
|
#endif /* !TC_DPU_USE_SCMI_CLK */
|
|
|
|
#if TC_DPU_USE_SIMPLE_PANEL
|
|
vpanel {
|
|
compatible = "panel-dpi";
|
|
post-init-providers = <&pl0>;
|
|
port {
|
|
lcd_in: endpoint {
|
|
remote-endpoint = <&dp_pl0_out0>;
|
|
};
|
|
};
|
|
|
|
panel-timing {
|
|
LCD_TIMING;
|
|
};
|
|
};
|
|
|
|
#else
|
|
vencoder {
|
|
compatible = "drm,virtual-encoder";
|
|
port {
|
|
lcd_in: endpoint {
|
|
remote-endpoint = <&dp_pl0_out0>;
|
|
};
|
|
};
|
|
|
|
display-timings {
|
|
timing-panel {
|
|
LCD_TIMING;
|
|
};
|
|
};
|
|
|
|
};
|
|
#endif
|
|
ethernet: ethernet@ETHERNET_ADDR {
|
|
reg = <0x0 ADDRESSIFY(ETHERNET_ADDR) 0x0 0x10000>;
|
|
interrupts = <GIC_SPI ETHERNET_INT IRQ_TYPE_LEVEL_HIGH 0>;
|
|
|
|
reg-io-width = <2>;
|
|
smsc,irq-push-pull;
|
|
};
|
|
|
|
bp_clock24mhz: clock24mhz {
|
|
compatible = "fixed-clock";
|
|
#clock-cells = <0>;
|
|
clock-frequency = <24000000>;
|
|
clock-output-names = "bp:clock24mhz";
|
|
};
|
|
|
|
sysreg: sysreg@SYS_REGS_ADDR {
|
|
compatible = "arm,vexpress-sysreg";
|
|
reg = <0x0 ADDRESSIFY(SYS_REGS_ADDR) 0x0 0x1000>;
|
|
gpio-controller;
|
|
#gpio-cells = <2>;
|
|
};
|
|
|
|
fixed_3v3: v2m-3v3 {
|
|
compatible = "regulator-fixed";
|
|
regulator-name = "3V3";
|
|
regulator-min-microvolt = <3300000>;
|
|
regulator-max-microvolt = <3300000>;
|
|
regulator-always-on;
|
|
};
|
|
|
|
mmci: mmci@MMC_ADDR {
|
|
compatible = "arm,pl180", "arm,primecell";
|
|
reg = <0x0 ADDRESSIFY(MMC_ADDR) 0x0 0x1000>;
|
|
interrupts = <GIC_SPI MMC_INT_0 IRQ_TYPE_LEVEL_HIGH 0>,
|
|
<GIC_SPI MMC_INT_1 IRQ_TYPE_LEVEL_HIGH 0>;
|
|
wp-gpios = <&sysreg 1 0>;
|
|
bus-width = <4>;
|
|
max-frequency = <25000000>;
|
|
vmmc-supply = <&fixed_3v3>;
|
|
clocks = <&bp_clock24mhz>, <&bp_clock24mhz>;
|
|
clock-names = "mclk", "apb_pclk";
|
|
};
|
|
|
|
gpu_clk: gpu_clk {
|
|
compatible = "fixed-clock";
|
|
#clock-cells = <0>;
|
|
clock-frequency = <1000000000>;
|
|
};
|
|
|
|
gpu_core_clk: gpu_core_clk {
|
|
compatible = "fixed-clock";
|
|
#clock-cells = <0>;
|
|
clock-frequency = <1000000000>;
|
|
};
|
|
|
|
gpu: gpu@2d000000 {
|
|
compatible = "arm,mali-midgard";
|
|
reg = <0x0 0x2d000000 0x0 0x200000>;
|
|
clocks = <&gpu_core_clk>;
|
|
clock-names = "shadercores";
|
|
#if TC_SCMI_PD_CTRL_EN
|
|
power-domains = <&scmi_devpd GPU_SCMI_PD_IDX>;
|
|
scmi-perf-domain = <3>;
|
|
#endif /* TC_SCMI_PD_CTRL_EN */
|
|
|
|
pbha {
|
|
int-id-override = <0 0x22>, <2 0x23>, <4 0x23>, <7 0x22>,
|
|
<8 0x22>, <9 0x22>, <10 0x22>, <11 0x22>,
|
|
<12 0x22>, <13 0x22>, <16 0x22>, <17 0x32>,
|
|
<18 0x32>, <19 0x22>, <20 0x22>, <21 0x32>,
|
|
<22 0x32>, <24 0x22>, <28 0x32>;
|
|
propagate-bits = <0x0f>;
|
|
};
|
|
};
|
|
|
|
power_model_simple {
|
|
/*
|
|
* Numbers used are irrelevant to Titan,
|
|
* it helps suppressing the kernel warnings.
|
|
*/
|
|
compatible = "arm,mali-simple-power-model";
|
|
static-coefficient = <2427750>;
|
|
dynamic-coefficient = <4687>;
|
|
ts = <20000 2000 (-20) 2>;
|
|
thermal-zone = "";
|
|
};
|
|
|
|
smmu_600: smmu@2ce00000 {
|
|
compatible = "arm,smmu-v3";
|
|
reg = <0 0x2ce00000 0 0x20000>;
|
|
interrupts = <GIC_SPI 75 IRQ_TYPE_EDGE_RISING 0>,
|
|
<GIC_SPI 74 IRQ_TYPE_EDGE_RISING 0>,
|
|
<GIC_SPI 76 IRQ_TYPE_EDGE_RISING 0>,
|
|
<GIC_SPI 77 IRQ_TYPE_EDGE_RISING 0>;
|
|
interrupt-names = "eventq", "priq", "cmdq-sync", "gerror";
|
|
#iommu-cells = <1>;
|
|
status = "disabled";
|
|
};
|
|
|
|
smmu_700: iommu@3f000000 {
|
|
#iommu-cells = <1>;
|
|
compatible = "arm,smmu-v3";
|
|
reg = <0x0 0x3f000000 0x0 0x5000000>;
|
|
interrupts = <GIC_SPI 228 IRQ_TYPE_EDGE_RISING 0>,
|
|
<GIC_SPI 229 IRQ_TYPE_EDGE_RISING 0>,
|
|
<GIC_SPI 230 IRQ_TYPE_EDGE_RISING 0>;
|
|
interrupt-names = "eventq", "cmdq-sync", "gerror";
|
|
dma-coherent;
|
|
status = "disabled";
|
|
};
|
|
|
|
smmu_700_dpu: iommu@4002a00000 {
|
|
#iommu-cells = <1>;
|
|
compatible = "arm,smmu-v3";
|
|
reg = <HI(0x4002a00000) LO(0x4002a00000) 0x0 0x5000000>;
|
|
interrupts = <GIC_SPI 481 IRQ_TYPE_EDGE_RISING 0>,
|
|
<GIC_SPI 482 IRQ_TYPE_EDGE_RISING 0>,
|
|
<GIC_SPI 483 IRQ_TYPE_EDGE_RISING 0>;
|
|
interrupt-names = "eventq", "cmdq-sync", "gerror";
|
|
dma-coherent;
|
|
status = "disabled";
|
|
};
|
|
|
|
dp0: display@DPU_ADDR {
|
|
#address-cells = <1>;
|
|
#size-cells = <0>;
|
|
compatible = "arm,mali-d71";
|
|
reg = <HI(ADDRESSIFY(DPU_ADDR)) LO(ADDRESSIFY(DPU_ADDR)) 0 0x20000>;
|
|
interrupts = <GIC_SPI DPU_IRQ IRQ_TYPE_LEVEL_HIGH 0>;
|
|
interrupt-names = "DPU";
|
|
DPU_CLK_ATTR1;
|
|
|
|
pl0: pipeline@0 {
|
|
reg = <0>;
|
|
DPU_CLK_ATTR2;
|
|
pl_id = <0>;
|
|
ports {
|
|
#address-cells = <1>;
|
|
#size-cells = <0>;
|
|
port@0 {
|
|
reg = <0>;
|
|
dp_pl0_out0: endpoint {
|
|
remote-endpoint = <&lcd_in>;
|
|
};
|
|
};
|
|
};
|
|
};
|
|
|
|
pl1: pipeline@1 {
|
|
reg = <1>;
|
|
DPU_CLK_ATTR3;
|
|
pl_id = <1>;
|
|
ports {
|
|
#address-cells = <1>;
|
|
#size-cells = <0>;
|
|
port@0 {
|
|
reg = <0>;
|
|
};
|
|
};
|
|
};
|
|
};
|
|
|
|
/*
|
|
* L3 cache in the DSU is the Memory System Component (MSC)
|
|
* The MPAM registers are accessed through utility bus in the DSU
|
|
*/
|
|
dsu-msc0 {
|
|
compatible = "arm,mpam-msc";
|
|
reg = <DSU_MPAM_ADDR 0x0 0x2000>;
|
|
};
|
|
|
|
ete0 {
|
|
compatible = "arm,embedded-trace-extension";
|
|
cpu = <&CPU0>;
|
|
};
|
|
|
|
ete1 {
|
|
compatible = "arm,embedded-trace-extension";
|
|
cpu = <&CPU1>;
|
|
};
|
|
|
|
ete2 {
|
|
compatible = "arm,embedded-trace-extension";
|
|
cpu = <&CPU2>;
|
|
};
|
|
|
|
ete3 {
|
|
compatible = "arm,embedded-trace-extension";
|
|
cpu = <&CPU3>;
|
|
};
|
|
|
|
ete4 {
|
|
compatible = "arm,embedded-trace-extension";
|
|
cpu = <&CPU4>;
|
|
};
|
|
|
|
ete5 {
|
|
compatible = "arm,embedded-trace-extension";
|
|
cpu = <&CPU5>;
|
|
};
|
|
|
|
ete6 {
|
|
compatible = "arm,embedded-trace-extension";
|
|
cpu = <&CPU6>;
|
|
};
|
|
|
|
ete7 {
|
|
compatible = "arm,embedded-trace-extension";
|
|
cpu = <&CPU7>;
|
|
};
|
|
|
|
trbe {
|
|
compatible = "arm,trace-buffer-extension";
|
|
interrupts = <GIC_PPI 2 IRQ_TYPE_LEVEL_LOW 0>;
|
|
};
|
|
|
|
trusty {
|
|
#size-cells = <0x02>;
|
|
#address-cells = <0x02>;
|
|
ranges = <0x00>;
|
|
compatible = "android,trusty-v1";
|
|
|
|
virtio {
|
|
compatible = "android,trusty-virtio-v1";
|
|
};
|
|
|
|
test {
|
|
compatible = "android,trusty-test-v1";
|
|
};
|
|
|
|
log {
|
|
compatible = "android,trusty-log-v1";
|
|
};
|
|
|
|
irq {
|
|
ipi-range = <0x08 0x0f 0x08>;
|
|
interrupt-ranges = <0x00 0x0f 0x00 0x10 0x1f 0x01 0x20 0x3f 0x02>;
|
|
interrupt-templates = <0x01 0x00 0x8001 0x01 0x01 0x04 0x8001 0x01 0x00 0x04>;
|
|
compatible = "android,trusty-irq-v1";
|
|
};
|
|
};
|
|
|
|
/* used in U-boot, Linux doesn't care */
|
|
arm_ffa {
|
|
compatible = "arm,ffa";
|
|
method = "smc";
|
|
};
|
|
};
|