mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-15 00:54:22 +00:00
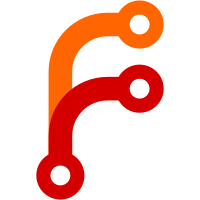
The simplistic view of a core's powerdown sequence is that power is atomically cut upon calling `wfi`. However, it turns out that it has lots to do - it has to talk to the interconnect to exit coherency, clean caches, check for RAS errors, etc. These take significant amounts of time and are certainly not atomic. As such there is a significant window of opportunity for external events to happen. Many of these steps are not destructive to context, so theoretically, the core can just "give up" half way (or roll certain actions back) and carry on running. The point in this sequence after which roll back is not possible is called the point of no return. One of these actions is the checking for RAS errors. It is possible for one to happen during this lengthy sequence, or at least remain undiscovered until that point. If the core were to continue powerdown when that happens, there would be no (easy) way to inform anyone about it. Rejecting the powerdown and letting software handle the error is the best way to implement this. Arm cores since at least the a510 have included this exact feature. So far it hasn't been deemed necessary to account for it in firmware due to the low likelihood of this happening. However, events like GIC wakeup requests are much more probable. Older cores will powerdown and immediately power back up when this happens. Travis and Gelas include a feature similar to the RAS case above, called powerdown abandon. The idea is that this will improve the latency to service the interrupt by saving on work which the core and software need to do. So far firmware has relied on the `wfi` being the point of no return and if it doesn't explicitly detect a pending interrupt quite early on, it will embark onto a sequence that it expects to end with shutdown. To accommodate for it not being a point of no return, we must undo all of the system management we did, just like in the warm boot entrypoint. To achieve that, the pwr_domain_pwr_down_wfi hook must not be terminal. Most recent platforms do some platform management and finish on the standard `wfi`, followed by a panic or an endless loop as this is expected to not return. To make this generic, any platform that wishes to support wakeups must instead let common code call `psci_power_down_wfi()` right after. Besides wakeups, this lets common code handle powerdown errata better as well. Then, the CPU_OFF case is simple - PSCI does not allow it to return. So the best that can be done is to attempt the `wfi` a few times (the choice of 32 is arbitrary) in the hope that the wakeup is transient. If it isn't, the only choice is to panic, as the system is likely to be in a bad state, eg. interrupts weren't routed away. The same applies for SYSTEM_OFF, SYSTEM_RESET, and SYSTEM_RESET2. There the panic won't matter as the system is going offline one way or another. The RAS case will be considered in a separate patch. Now, the CPU_SUSPEND case is more involved. First, to powerdown it must wipe its context as it is not written on warm boot. But it cannot be overwritten in case of a wakeup. To avoid the catch 22, save a copy that will only be used if powerdown fails. That is about 500 bytes on the stack so it hopefully doesn't tip anyone over any limits. In future that can be avoided by having a core manage its own context. Second, when the core wakes up, it must undo anything it did to prepare for poweroff, which for the cores we care about, is writing CPUPWRCTLR_EL1.CORE_PWRDN_EN. The least intrusive for the cpu library way of doing this is to simply call the power off hook again and have the hook toggle the bit. If in the future there need to be more complex sequences, their direction can be advised on the value of this bit. Third, do the actual "resume". Most of the logic is already there for the retention suspend, so that only needs a small touch up to apply to the powerdown case as well. The missing bit is the powerdown specific state management. Luckily, the warmboot entrypoint does exactly that already too, so steal that and we're done. All of this is hidden behind a FEAT_PABANDON flag since it has a large memory and runtime cost that we don't want to burden non pabandon cores with. Finally, do some function renaming to better reflect their purpose and make names a little bit more consistent. Change-Id: I2405b59300c2e24ce02e266f91b7c51474c1145f Signed-off-by: Boyan Karatotev <boyan.karatotev@arm.com>
88 lines
2 KiB
C
88 lines
2 KiB
C
/*
|
|
* Copyright (c) 2014-2024, Arm Limited and Contributors. All rights reserved.
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
|
|
#include <assert.h>
|
|
#include <stddef.h>
|
|
|
|
#include <arch_helpers.h>
|
|
#include <common/debug.h>
|
|
#include <drivers/console.h>
|
|
#include <plat/common/platform.h>
|
|
|
|
#include "psci_private.h"
|
|
|
|
void __dead2 psci_system_off(void)
|
|
{
|
|
psci_print_power_domain_map();
|
|
|
|
assert(psci_plat_pm_ops->system_off != NULL);
|
|
|
|
/* Notify the Secure Payload Dispatcher */
|
|
if ((psci_spd_pm != NULL) && (psci_spd_pm->svc_system_off != NULL)) {
|
|
psci_spd_pm->svc_system_off();
|
|
}
|
|
|
|
console_flush();
|
|
|
|
/* Call the platform specific hook */
|
|
psci_plat_pm_ops->system_off();
|
|
|
|
psci_pwrdown_cpu_end_terminal();
|
|
}
|
|
|
|
void __dead2 psci_system_reset(void)
|
|
{
|
|
psci_print_power_domain_map();
|
|
|
|
assert(psci_plat_pm_ops->system_reset != NULL);
|
|
|
|
/* Notify the Secure Payload Dispatcher */
|
|
if ((psci_spd_pm != NULL) && (psci_spd_pm->svc_system_reset != NULL)) {
|
|
psci_spd_pm->svc_system_reset();
|
|
}
|
|
|
|
console_flush();
|
|
|
|
/* Call the platform specific hook */
|
|
psci_plat_pm_ops->system_reset();
|
|
|
|
psci_pwrdown_cpu_end_terminal();
|
|
}
|
|
|
|
u_register_t psci_system_reset2(uint32_t reset_type, u_register_t cookie)
|
|
{
|
|
unsigned int is_vendor;
|
|
|
|
psci_print_power_domain_map();
|
|
|
|
assert(psci_plat_pm_ops->system_reset2 != NULL);
|
|
|
|
is_vendor = (reset_type >> PSCI_RESET2_TYPE_VENDOR_SHIFT) & 1U;
|
|
if (is_vendor == 0U) {
|
|
/*
|
|
* Only WARM_RESET is allowed for architectural type resets.
|
|
*/
|
|
if (reset_type != PSCI_RESET2_SYSTEM_WARM_RESET)
|
|
return (u_register_t) PSCI_E_INVALID_PARAMS;
|
|
if ((psci_plat_pm_ops->write_mem_protect != NULL) &&
|
|
(psci_plat_pm_ops->write_mem_protect(0) < 0)) {
|
|
return (u_register_t) PSCI_E_NOT_SUPPORTED;
|
|
}
|
|
}
|
|
|
|
/* Notify the Secure Payload Dispatcher */
|
|
if ((psci_spd_pm != NULL) && (psci_spd_pm->svc_system_reset != NULL)) {
|
|
psci_spd_pm->svc_system_reset();
|
|
}
|
|
console_flush();
|
|
|
|
u_register_t ret =
|
|
(u_register_t) psci_plat_pm_ops->system_reset2((int) is_vendor, reset_type, cookie);
|
|
if (ret != PSCI_E_SUCCESS)
|
|
return ret;
|
|
|
|
psci_pwrdown_cpu_end_terminal();
|
|
}
|