mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-08 05:43:53 +00:00
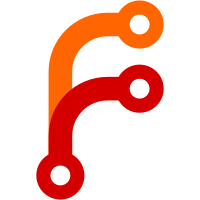
Errata application is painful for performance. For a start, it's done when the core has just come out of reset, which means branch predictors and caches will be empty so a branch to a workaround function must be fetched from memory and that round trip is very slow. Then it also runs with the I-cache off, which means that the loop to iterate over the workarounds must also be fetched from memory on each iteration. We can remove both branches. First, we can simply apply every erratum directly instead of defining a workaround function and jumping to it. Currently, no errata that need to be applied at both reset and runtime, with the same workaround function, exist. If the need arose in future, this should be achievable with a reset + runtime wrapper combo. Then, we can construct a function that applies each erratum linearly instead of looping over the list. If this function is part of the reset function, then the only "far" branches at reset will be for the checker functions. Importantly, this mitigates the slowdown even when an erratum is disabled. The result is ~50% speedup on N1SDP and ~20% on AArch64 Juno on wakeup from PSCI calls that end in powerdown. This is roughly back to the baseline of v2.9, before the errata framework regressed on performance (or a little better). It is important to note that there are other slowdowns since then that remain unknown. Change-Id: Ie4d5288a331b11fd648e5c4a0b652b74160b07b9 Signed-off-by: Boyan Karatotev <boyan.karatotev@arm.com>
109 lines
2.9 KiB
ArmAsm
109 lines
2.9 KiB
ArmAsm
/*
|
|
* Copyright (c) 2014-2025, Arm Limited and Contributors. All rights reserved.
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
#include <aem_generic.h>
|
|
#include <arch.h>
|
|
#include <asm_macros.S>
|
|
#include <cpu_macros.S>
|
|
|
|
cpu_reset_prologue aem_generic
|
|
|
|
func aem_generic_core_pwr_dwn
|
|
/* ---------------------------------------------
|
|
* Disable the Data Cache.
|
|
* ---------------------------------------------
|
|
*/
|
|
mrs x1, sctlr_el3
|
|
bic x1, x1, #SCTLR_C_BIT
|
|
msr sctlr_el3, x1
|
|
isb
|
|
|
|
/* ---------------------------------------------
|
|
* AEM model supports L3 caches in which case L2
|
|
* will be private per core caches and flush
|
|
* from L1 to L2 is not sufficient.
|
|
* ---------------------------------------------
|
|
*/
|
|
mrs x1, clidr_el1
|
|
|
|
/* ---------------------------------------------
|
|
* Check if L3 cache is implemented.
|
|
* ---------------------------------------------
|
|
*/
|
|
tst x1, ((1 << CLIDR_FIELD_WIDTH) - 1) << CTYPE_SHIFT(3)
|
|
|
|
/* ---------------------------------------------
|
|
* There is no L3 cache, flush L1 to L2 only.
|
|
* ---------------------------------------------
|
|
*/
|
|
mov x0, #DCCISW
|
|
b.eq dcsw_op_level1
|
|
|
|
mov x18, x30
|
|
|
|
/* ---------------------------------------------
|
|
* Flush L1 cache to L2.
|
|
* ---------------------------------------------
|
|
*/
|
|
bl dcsw_op_level1
|
|
mov x30, x18
|
|
|
|
/* ---------------------------------------------
|
|
* Flush L2 cache to L3.
|
|
* ---------------------------------------------
|
|
*/
|
|
mov x0, #DCCISW
|
|
b dcsw_op_level2
|
|
endfunc aem_generic_core_pwr_dwn
|
|
|
|
func aem_generic_cluster_pwr_dwn
|
|
/* ---------------------------------------------
|
|
* Disable the Data Cache.
|
|
* ---------------------------------------------
|
|
*/
|
|
mrs x1, sctlr_el3
|
|
bic x1, x1, #SCTLR_C_BIT
|
|
msr sctlr_el3, x1
|
|
isb
|
|
|
|
/* ---------------------------------------------
|
|
* Flush all caches to PoC.
|
|
* ---------------------------------------------
|
|
*/
|
|
mov x0, #DCCISW
|
|
b dcsw_op_all
|
|
endfunc aem_generic_cluster_pwr_dwn
|
|
|
|
cpu_reset_func_start aem_generic
|
|
cpu_reset_func_end aem_generic
|
|
|
|
/* ---------------------------------------------
|
|
* This function provides cpu specific
|
|
* register information for crash reporting.
|
|
* It needs to return with x6 pointing to
|
|
* a list of register names in ascii and
|
|
* x8 - x15 having values of registers to be
|
|
* reported.
|
|
* ---------------------------------------------
|
|
*/
|
|
.section .rodata.aem_generic_regs, "aS"
|
|
aem_generic_regs: /* The ascii list of register names to be reported */
|
|
.asciz "" /* no registers to report */
|
|
|
|
func aem_generic_cpu_reg_dump
|
|
adr x6, aem_generic_regs
|
|
ret
|
|
endfunc aem_generic_cpu_reg_dump
|
|
|
|
|
|
/* cpu_ops for Base AEM FVP */
|
|
declare_cpu_ops aem_generic, BASE_AEM_MIDR, aem_generic_reset_func, \
|
|
aem_generic_core_pwr_dwn, \
|
|
aem_generic_cluster_pwr_dwn
|
|
|
|
/* cpu_ops for Foundation FVP */
|
|
declare_cpu_ops aem_generic, FOUNDATION_AEM_MIDR, aem_generic_reset_func, \
|
|
aem_generic_core_pwr_dwn, \
|
|
aem_generic_cluster_pwr_dwn
|