mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-19 02:54:24 +00:00
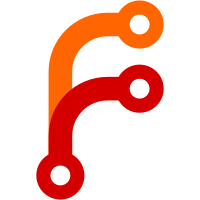
Change-Id: I4f58bb4660078c9bc76d2826c90b2fa711719a3e Signed-off-by: Antonio Nino Diaz <antonio.ninodiaz@arm.com>
48 lines
1,021 B
C
48 lines
1,021 B
C
/*
|
|
* Copyright (c) 2012-2017 Roberto E. Vargas Caballero
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
/*
|
|
* Portions copyright (c) 2018, ARM Limited and Contributors.
|
|
* All rights reserved.
|
|
*/
|
|
|
|
#ifndef _TIME_H
|
|
#define _TIME_H
|
|
|
|
#include <time_.h>
|
|
|
|
#ifndef NULL
|
|
#define NULL ((void *) 0)
|
|
#endif
|
|
|
|
#define CLOCKS_PER_SEC 1000000
|
|
|
|
typedef long int clock_t;
|
|
|
|
struct tm {
|
|
int tm_sec;
|
|
int tm_min;
|
|
int tm_hour;
|
|
int tm_mday;
|
|
int tm_mon;
|
|
int tm_year;
|
|
int tm_wday;
|
|
int tm_yday;
|
|
int tm_isdst;
|
|
};
|
|
|
|
extern clock_t clock(void);
|
|
extern double difftime(time_t time1, time_t time0);
|
|
extern time_t mktime(struct tm *timeptr);
|
|
extern time_t time(time_t *timer);
|
|
extern char *asctime(const struct tm *timeptr);
|
|
extern char *ctime(const time_t *timer);
|
|
extern struct tm *gmtime(const time_t *timer);
|
|
extern struct tm *localtime(const time_t *timer);
|
|
extern size_t strftime(char * restrict s, size_t maxsize,
|
|
const char * restrict format,
|
|
const struct tm * restrict timeptr);
|
|
|
|
#endif
|