mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-04-15 09:04:17 +00:00
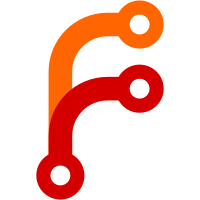
This patch reworks the cold boot path across the BL1, BL2, BL3-1 and BL3-2 boot loader stages to not use stacks allocated in coherent memory for early platform setup and enabling the MMU. Stacks allocated in normal memory are used instead. Attributes for stack memory change from nGnRnE when the MMU is disabled to Normal WBWA Inner-shareable when the MMU and data cache are enabled. It is possible for the CPU to read stale stack memory after the MMU is enabled from another CPUs cache. Hence, it is unsafe to turn on the MMU and data cache while using normal stacks when multiple CPUs are a part of the same coherency domain. It is safe to do so in the cold boot path as only the primary cpu executes it. The secondary cpus are in a quiescent state. This patch does not remove the allocation of coherent stack memory. That is done in a subsequent patch. Change-Id: I12c80b7c7ab23506d425c5b3a8a7de693498f830
190 lines
6.1 KiB
ArmAsm
190 lines
6.1 KiB
ArmAsm
/*
|
|
* Copyright (c) 2013-2014, ARM Limited and Contributors. All rights reserved.
|
|
*
|
|
* Redistribution and use in source and binary forms, with or without
|
|
* modification, are permitted provided that the following conditions are met:
|
|
*
|
|
* Redistributions of source code must retain the above copyright notice, this
|
|
* list of conditions and the following disclaimer.
|
|
*
|
|
* Redistributions in binary form must reproduce the above copyright notice,
|
|
* this list of conditions and the following disclaimer in the documentation
|
|
* and/or other materials provided with the distribution.
|
|
*
|
|
* Neither the name of ARM nor the names of its contributors may be used
|
|
* to endorse or promote products derived from this software without specific
|
|
* prior written permission.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
|
|
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
|
|
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
|
|
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
|
|
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
|
|
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
|
|
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
|
|
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
|
|
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
|
|
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
|
|
* POSSIBILITY OF SUCH DAMAGE.
|
|
*/
|
|
|
|
#include <arch.h>
|
|
#include <asm_macros.S>
|
|
#include <bl_common.h>
|
|
|
|
.globl bl31_entrypoint
|
|
|
|
|
|
/* -----------------------------------------------------
|
|
* bl31_entrypoint() is the cold boot entrypoint,
|
|
* executed only by the primary cpu.
|
|
* -----------------------------------------------------
|
|
*/
|
|
|
|
func bl31_entrypoint
|
|
/* ---------------------------------------------------------------
|
|
* Preceding bootloader has populated x0 with a pointer to a
|
|
* 'bl31_params' structure & x1 with a pointer to platform
|
|
* specific structure
|
|
* ---------------------------------------------------------------
|
|
*/
|
|
#if !RESET_TO_BL31
|
|
mov x20, x0
|
|
mov x21, x1
|
|
#else
|
|
|
|
/* -----------------------------------------------------
|
|
* Perform any processor specific actions upon reset
|
|
* e.g. cache, tlb invalidations etc. Override the
|
|
* Boot ROM(BL0) programming sequence
|
|
* -----------------------------------------------------
|
|
*/
|
|
bl cpu_reset_handler
|
|
#endif
|
|
|
|
/* ---------------------------------------------
|
|
* Enable the instruction cache.
|
|
* ---------------------------------------------
|
|
*/
|
|
mrs x1, sctlr_el3
|
|
orr x1, x1, #SCTLR_I_BIT
|
|
msr sctlr_el3, x1
|
|
isb
|
|
|
|
/* ---------------------------------------------
|
|
* Set the exception vector and zero tpidr_el3
|
|
* until the crash reporting is set up
|
|
* ---------------------------------------------
|
|
*/
|
|
adr x1, runtime_exceptions
|
|
msr vbar_el3, x1
|
|
msr tpidr_el3, xzr
|
|
|
|
/* ---------------------------------------------------------------------
|
|
* The initial state of the Architectural feature trap register
|
|
* (CPTR_EL3) is unknown and it must be set to a known state. All
|
|
* feature traps are disabled. Some bits in this register are marked as
|
|
* Reserved and should not be modified.
|
|
*
|
|
* CPTR_EL3.TCPAC: This causes a direct access to the CPACR_EL1 from EL1
|
|
* or the CPTR_EL2 from EL2 to trap to EL3 unless it is trapped at EL2.
|
|
* CPTR_EL3.TTA: This causes access to the Trace functionality to trap
|
|
* to EL3 when executed from EL0, EL1, EL2, or EL3. If system register
|
|
* access to trace functionality is not supported, this bit is RES0.
|
|
* CPTR_EL3.TFP: This causes instructions that access the registers
|
|
* associated with Floating Point and Advanced SIMD execution to trap
|
|
* to EL3 when executed from any exception level, unless trapped to EL1
|
|
* or EL2.
|
|
* ---------------------------------------------------------------------
|
|
*/
|
|
mrs x1, cptr_el3
|
|
bic w1, w1, #TCPAC_BIT
|
|
bic w1, w1, #TTA_BIT
|
|
bic w1, w1, #TFP_BIT
|
|
msr cptr_el3, x1
|
|
|
|
#if RESET_TO_BL31
|
|
/* -------------------------------------------------------
|
|
* Will not return from this macro if it is a warm boot.
|
|
* -------------------------------------------------------
|
|
*/
|
|
wait_for_entrypoint
|
|
bl platform_mem_init
|
|
#else
|
|
/* ---------------------------------------------
|
|
* This is BL31 which is expected to be executed
|
|
* only by the primary cpu (at least for now).
|
|
* So, make sure no secondary has lost its way.
|
|
* ---------------------------------------------
|
|
*/
|
|
mrs x0, mpidr_el1
|
|
bl platform_is_primary_cpu
|
|
cbz x0, _panic
|
|
#endif
|
|
|
|
/* ---------------------------------------------
|
|
* Zero out NOBITS sections. There are 2 of them:
|
|
* - the .bss section;
|
|
* - the coherent memory section.
|
|
* ---------------------------------------------
|
|
*/
|
|
ldr x0, =__BSS_START__
|
|
ldr x1, =__BSS_SIZE__
|
|
bl zeromem16
|
|
|
|
ldr x0, =__COHERENT_RAM_START__
|
|
ldr x1, =__COHERENT_RAM_UNALIGNED_SIZE__
|
|
bl zeromem16
|
|
|
|
/* ---------------------------------------------
|
|
* Initialise cpu_data and crash reporting
|
|
* ---------------------------------------------
|
|
*/
|
|
#if CRASH_REPORTING
|
|
bl init_crash_reporting
|
|
#endif
|
|
bl init_cpu_data_ptr
|
|
|
|
/* ---------------------------------------------
|
|
* Use SP_EL0 for the C runtime stack.
|
|
* ---------------------------------------------
|
|
*/
|
|
msr spsel, #0
|
|
|
|
/* --------------------------------------------
|
|
* Allocate a stack whose memory will be marked
|
|
* as Normal-IS-WBWA when the MMU is enabled.
|
|
* There is no risk of reading stale stack
|
|
* memory after enabling the MMU as only the
|
|
* primary cpu is running at the moment.
|
|
* --------------------------------------------
|
|
*/
|
|
mrs x0, mpidr_el1
|
|
bl platform_set_stack
|
|
|
|
/* ---------------------------------------------
|
|
* Perform platform specific early arch. setup
|
|
* ---------------------------------------------
|
|
*/
|
|
#if RESET_TO_BL31
|
|
mov x0, 0
|
|
mov x1, 0
|
|
#else
|
|
mov x0, x20
|
|
mov x1, x21
|
|
#endif
|
|
|
|
bl bl31_early_platform_setup
|
|
bl bl31_plat_arch_setup
|
|
|
|
/* ---------------------------------------------
|
|
* Jump to main function.
|
|
* ---------------------------------------------
|
|
*/
|
|
bl bl31_main
|
|
|
|
b el3_exit
|
|
|
|
_panic:
|
|
wfi
|
|
b _panic
|