mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-05-01 08:05:46 +00:00
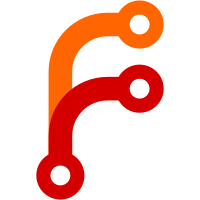
More often than not, Arm based systems include some revision of a GIC.
There are two ways of adding support for them in platform code - calling
the top-level helpers from plat/arm/common/arm_gicvX.c or by using the
driver directly. Both of these methods allow for a high degree of
customisation - most functions are defined to be weak and there are no
calls to any of them in generic code.
As it turns out, requirements around those GICs are largely the same.
Platforms that use arm_gicvX.c use the helpers identically among each
other. Platforms that use the driver directly tend to end up with calls
that look a lot like the arm_gicvX.c helpers and the weakness of the
functions are never exercised.
All of this results in a lot of code duplication to do what is
essentially the same thing. Even though it's not a lot of code, when
multiplied among many platforms it becomes significant and makes
refactoring it quite difficult. It's also bug prone since the steps are
a little convoluted and things are likely to work even with subtle
errors (see 50009f6117
).
So promote as much of the GIC to be called from common code. Do the
setup in bl31_main() and have every PSCI method do the state management
directly instead of delegating it to the platform hooks. We can base
this implementation on arm_gicvX.c since they already offer logical
names and have worked quite well so far with minimal changes.
The main benefit of doing this is reduced code duplication. If we assume
that, outside of some platform setup, GIC management is identical, then
a platform can add support by telling the build system, regardless of
GIC revision. The other benefit is performance - BL31 and PSCI already
know the core_pos and they can pass it as an argument instead of having
to call plat_my_core_pos(). Now, the only platform specific GIC actions
necessary are the saving and restoring of context on entering and
exiting a power domain. The PSCI library does not keep track of this so
it is unable perform it itself. The routines themselves are also
provided.
For compatibility all of this is hidden behind a build flag. Platforms
are encouraged to adopt this driver, but it would not be practical to
convert and validate every GIC based platform.
This patch renames the functions in question to follow the
gic_<function>() convention. This allows the names to be version
agnostic.
Finally, drop the weak definitions - they are unused, likely to remain
so, and can be added back if the need arises.
Change-Id: I5b5267f4b72f633fb1096400ec8e4b208694135f
Signed-off-by: Boyan Karatotev <boyan.karatotev@arm.com>
105 lines
2.5 KiB
C
105 lines
2.5 KiB
C
/*
|
|
* Copyright (c) 2014-2025, Arm Limited and Contributors. All rights reserved.
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
|
|
#include <assert.h>
|
|
#include <stddef.h>
|
|
|
|
#include <arch_helpers.h>
|
|
#include <common/debug.h>
|
|
#include <drivers/arm/gic.h>
|
|
#include <drivers/console.h>
|
|
#include <plat/common/platform.h>
|
|
|
|
#include "psci_private.h"
|
|
|
|
void __dead2 psci_system_off(void)
|
|
{
|
|
psci_print_power_domain_map();
|
|
|
|
assert(psci_plat_pm_ops->system_off != NULL);
|
|
|
|
/* Notify the Secure Payload Dispatcher */
|
|
if ((psci_spd_pm != NULL) && (psci_spd_pm->svc_system_off != NULL)) {
|
|
psci_spd_pm->svc_system_off();
|
|
}
|
|
|
|
console_flush();
|
|
|
|
#if USE_GIC_DRIVER
|
|
/* turn the GIC off before we hand off to the platform */
|
|
gic_cpuif_disable(plat_my_core_pos());
|
|
#endif /* USE_GIC_DRIVER */
|
|
|
|
/* Call the platform specific hook */
|
|
psci_plat_pm_ops->system_off();
|
|
|
|
psci_pwrdown_cpu_end_terminal();
|
|
}
|
|
|
|
void __dead2 psci_system_reset(void)
|
|
{
|
|
psci_print_power_domain_map();
|
|
|
|
assert(psci_plat_pm_ops->system_reset != NULL);
|
|
|
|
/* Notify the Secure Payload Dispatcher */
|
|
if ((psci_spd_pm != NULL) && (psci_spd_pm->svc_system_reset != NULL)) {
|
|
psci_spd_pm->svc_system_reset();
|
|
}
|
|
|
|
console_flush();
|
|
|
|
#if USE_GIC_DRIVER
|
|
/* turn the GIC off before we hand off to the platform */
|
|
gic_cpuif_disable(plat_my_core_pos());
|
|
#endif /* USE_GIC_DRIVER */
|
|
|
|
/* Call the platform specific hook */
|
|
psci_plat_pm_ops->system_reset();
|
|
|
|
psci_pwrdown_cpu_end_terminal();
|
|
}
|
|
|
|
u_register_t psci_system_reset2(uint32_t reset_type, u_register_t cookie)
|
|
{
|
|
unsigned int is_vendor;
|
|
|
|
psci_print_power_domain_map();
|
|
|
|
assert(psci_plat_pm_ops->system_reset2 != NULL);
|
|
|
|
is_vendor = (reset_type >> PSCI_RESET2_TYPE_VENDOR_SHIFT) & 1U;
|
|
if (is_vendor == 0U) {
|
|
/*
|
|
* Only WARM_RESET is allowed for architectural type resets.
|
|
*/
|
|
if (reset_type != PSCI_RESET2_SYSTEM_WARM_RESET) {
|
|
return (u_register_t) PSCI_E_INVALID_PARAMS;
|
|
}
|
|
if ((psci_plat_pm_ops->write_mem_protect != NULL) &&
|
|
(psci_plat_pm_ops->write_mem_protect(0) < 0)) {
|
|
return (u_register_t) PSCI_E_NOT_SUPPORTED;
|
|
}
|
|
}
|
|
|
|
/* Notify the Secure Payload Dispatcher */
|
|
if ((psci_spd_pm != NULL) && (psci_spd_pm->svc_system_reset != NULL)) {
|
|
psci_spd_pm->svc_system_reset();
|
|
}
|
|
console_flush();
|
|
|
|
#if USE_GIC_DRIVER
|
|
/* turn the GIC off before we hand off to the platform */
|
|
gic_cpuif_disable(plat_my_core_pos());
|
|
#endif /* USE_GIC_DRIVER */
|
|
|
|
u_register_t ret =
|
|
(u_register_t) psci_plat_pm_ops->system_reset2((int) is_vendor, reset_type, cookie);
|
|
if (ret != PSCI_E_SUCCESS)
|
|
return ret;
|
|
|
|
psci_pwrdown_cpu_end_terminal();
|
|
}
|