mirror of
https://github.com/ARM-software/arm-trusted-firmware.git
synced 2025-05-03 17:38:39 +00:00
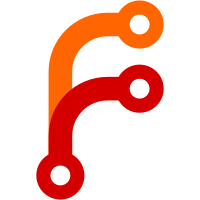
Building the default upstream configuration for the imx8mq-evk is no longer
possible: The linker will complain that the TF-A image will no longer
fit On-Chip SRAM.
In order to make the i.MX8MQ Image buildable again, let's make the DRAM
retention feature optional: It was added in v2.9 and it's possible to
boot the systems without it. Users that make space elsewhere and wish to
enable it can use the newly introduced IMX_DRAM_RETENTION parameter to
configure it. The parameter is added to all i.MX8M variants, but only
for i.MX8MQ, we disable it by default, as that's the one that currently
has binary size problems.
Change-Id: I714f8ea96f18154db02390ba500f4a2dc5329ee7
Fixes: dd108c3c1f
("feat(imx8mq): add the dram retention support for imx8mq")
Signed-off-by: Ahmad Fatoum <a.fatoum@pengutronix.de>
93 lines
2.2 KiB
C
93 lines
2.2 KiB
C
/*
|
|
* Copyright 2019-2023 NXP
|
|
*
|
|
* SPDX-License-Identifier: BSD-3-Clause
|
|
*/
|
|
|
|
#ifndef DRAM_H
|
|
#define DRAM_H
|
|
|
|
#include <assert.h>
|
|
|
|
#include <arch_helpers.h>
|
|
#include <lib/utils_def.h>
|
|
|
|
#include <ddrc.h>
|
|
#include <platform_def.h>
|
|
|
|
#define DDRC_LPDDR4 BIT(5)
|
|
#define DDRC_DDR4 BIT(4)
|
|
#define DDRC_DDR3L BIT(0)
|
|
#define DDR_TYPE_MASK U(0x3f)
|
|
#define ACTIVE_RANK_MASK U(0x3)
|
|
#define DDRC_ACTIVE_ONE_RANK U(0x1)
|
|
#define DDRC_ACTIVE_TWO_RANK U(0x2)
|
|
|
|
#define MR12 U(12)
|
|
#define MR14 U(14)
|
|
|
|
#define MAX_FSP_NUM U(3)
|
|
|
|
/* reg & config param */
|
|
struct dram_cfg_param {
|
|
unsigned int reg;
|
|
unsigned int val;
|
|
};
|
|
|
|
struct dram_timing_info {
|
|
/* umctl2 config */
|
|
struct dram_cfg_param *ddrc_cfg;
|
|
unsigned int ddrc_cfg_num;
|
|
/* ddrphy config */
|
|
struct dram_cfg_param *ddrphy_cfg;
|
|
unsigned int ddrphy_cfg_num;
|
|
/* ddr fsp train info */
|
|
struct dram_fsp_msg *fsp_msg;
|
|
unsigned int fsp_msg_num;
|
|
/* ddr phy trained CSR */
|
|
struct dram_cfg_param *ddrphy_trained_csr;
|
|
unsigned int ddrphy_trained_csr_num;
|
|
/* ddr phy PIE */
|
|
struct dram_cfg_param *ddrphy_pie;
|
|
unsigned int ddrphy_pie_num;
|
|
/* initialized fsp table */
|
|
unsigned int fsp_table[4];
|
|
};
|
|
|
|
struct dram_info {
|
|
int dram_type;
|
|
unsigned int num_rank;
|
|
uint32_t num_fsp;
|
|
int current_fsp;
|
|
int boot_fsp;
|
|
bool bypass_mode;
|
|
struct dram_timing_info *timing_info;
|
|
/* mr, emr, emr2, emr3, mr11, mr12, mr22, mr14 */
|
|
uint32_t mr_table[3][8];
|
|
/* used for workaround for rank to rank issue */
|
|
uint32_t rank_setting[3][3];
|
|
};
|
|
|
|
extern struct dram_info dram_info;
|
|
|
|
void dram_umctl2_init(struct dram_timing_info *timing);
|
|
void dram_phy_init(struct dram_timing_info *timing);
|
|
|
|
/* dram retention */
|
|
#if IMX_DRAM_RETENTION
|
|
void dram_info_init(unsigned long dram_timing_base);
|
|
void dram_enter_retention(void);
|
|
void dram_exit_retention(void);
|
|
#else
|
|
static inline void dram_info_init(unsigned long dram_timing_base) {}
|
|
static inline void dram_enter_retention(void) {}
|
|
static inline void dram_exit_retention(void) {}
|
|
#endif
|
|
|
|
void dram_clock_switch(unsigned int target_drate, bool bypass_mode);
|
|
|
|
/* dram frequency change */
|
|
void lpddr4_swffc(struct dram_info *info, unsigned int init_fsp, unsigned int fsp_index);
|
|
void ddr4_swffc(struct dram_info *dram_info, unsigned int pstate);
|
|
|
|
#endif /* DRAM_H */
|